listview实现查询功能
时间: 2023-10-16 11:18:02 浏览: 151
要实现ListView的查询功能,需要以下步骤:
1. 在布局文件中添加一个搜索框和一个ListView。
2. 在Java代码中,初始化ListView并将其与适配器绑定。
3. 实现搜索框的监听器,在用户输入时更新适配器中的数据。
以下是一个简单的示例代码:
1. 在布局文件中添加搜索框和ListView
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<SearchView
android:id="@+id/search_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:queryHint="Search"/>
<ListView
android:id="@+id/list_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
```
2. 在Java代码中初始化ListView并将其与适配器绑定。
```java
ListView listView = findViewById(R.id.list_view);
MyAdapter adapter = new MyAdapter(this, getData());
listView.setAdapter(adapter);
```
3. 实现搜索框的监听器,在用户输入时更新适配器中的数据。
```java
SearchView searchView = findViewById(R.id.search_view);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
adapter.filter(newText);
return true;
}
});
```
在适配器中,实现过滤方法来更新数据:
```java
public void filter(String text) {
data.clear();
if (TextUtils.isEmpty(text)) {
data.addAll(originalData);
} else {
text = text.toLowerCase();
for (Item item : originalData) {
if (item.getText().toLowerCase().contains(text)) {
data.add(item);
}
}
}
notifyDataSetChanged();
}
```
这样就可以实现ListView的查询功能了。
阅读全文
相关推荐
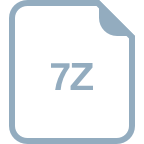
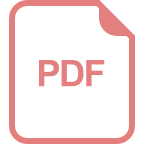
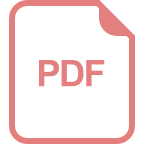















