代码解释:public static float[] projectedHistogram(final Mat img, Direction direction) { int sz = 0; switch (direction) { case HORIZONTAL: sz = img.rows(); break; case VERTICAL: sz = img.cols(); break; default: break; } // 统计这一行或一列中,非零元素的个数,并保存到nonZeroMat中 float[] nonZeroMat = new float[sz]; extractChannel(img, img, 0); for (int j = 0; j < sz; j++) { Mat data = (direction == Direction.HORIZONTAL) ? img.row(j) : img.col(j); int count = countNonZero(data); nonZeroMat[j] = count; } // Normalize histogram float max = 0; for (int j = 0; j < nonZeroMat.length; ++j) { max = Math.max(max, nonZeroMat[j]); } if (max > 0) { for (int j = 0; j < nonZeroMat.length; ++j) { nonZeroMat[j] /= max; } } return nonZeroMat; }
时间: 2024-04-04 14:35:19 浏览: 66
这段代码是一个计算图像投影直方图的方法。投影直方图是一种用于图像处理和计算机视觉的技术,用来描述图像中某个方向上像素值的分布情况。在这个方法中,输入参数img是一个Mat对象,表示输入的图像;direction是一个枚举类型,表示投影方向,可以是水平或垂直方向。
该方法首先根据投影方向确定投影直方图的尺寸,然后遍历图像的每一行或每一列,统计该行或该列中非零元素的个数,并将统计结果保存到一个float类型的数组nonZeroMat中。接着对直方图进行了归一化处理,即将直方图中每个元素的值除以最大值,使得所有元素的值都在[0,1]的范围内。
最后,该方法返回一个float类型的数组nonZeroMat,表示计算得到的投影直方图。
相关问题
代码解释:原理算法public static Mat features(final Mat in, final int sizeData) { float[] vhist = projectedHistogram(in, Direction.VERTICAL); float[] hhist = projectedHistogram(in, Direction.HORIZONTAL); Mat lowData = new Mat(); if (sizeData > 0) { resize(in, lowData, new Size(sizeData, sizeData)); } int numCols = vhist.length + hhist.length + lowData.cols() * lowData.rows(); Mat out = Mat.zeros(1, numCols, CV_32F).asMat(); FloatIndexer idx = out.createIndexer(); int j = 0; for (int i = 0; i < vhist.length; ++i, ++j) { idx.put(0, j, vhist[i]); } for (int i = 0; i < hhist.length; ++i, ++j) { idx.put(0, j, hhist[i]); } for (int x = 0; x < lowData.cols(); x++) { for (int y = 0; y < lowData.rows(); y++, ++j) { float val = lowData.ptr(x, y).get() & 0xFF; idx.put(0, j, val); } } return out; }
这段代码是字符识别算法中的一个函数,用于提取输入图像的特征。输入参数为一个Mat类型的图像in和一个整型参数sizeData,表示需要将图像缩放到的大小。该函数返回一个Mat类型的特征矩阵。
该函数的主要过程包括:
1. 调用projectedHistogram函数,分别对输入图像进行水平和垂直方向上的投影,得到一个长度为in.rows()+in.cols()的浮点型数组vhist和hhist,表示图像中每一行和每一列的像素值之和。
2. 如果sizeData大于0,调用resize函数,将输入图像缩放到sizeData*sizeData的大小,并保存到lowData中。
3. 计算特征矩阵的列数numCols,等于vhist和hhist的长度之和,再加上lowData的像素数。
4. 新建一个大小为1 x numCols的全零矩阵out,类型为CV_32F。
5. 使用out的FloatIndexer,将vhist和hhist的值依次存入out的第一行中。
6. 对于lowData中的每个像素,将其值存入out中。
7. 返回特征矩阵out。
需要注意的是,该函数依赖于projectedHistogram函数的实现,这个函数的具体实现没有给出。
代码解释具体算法public static Mat features(final Mat in, final int sizeData) { float[] vhist = projectedHistogram(in, Direction.VERTICAL); float[] hhist = projectedHistogram(in, Direction.HORIZONTAL); Mat lowData = new Mat(); if (sizeData > 0) { resize(in, lowData, new Size(sizeData, sizeData)); } int numCols = vhist.length + hhist.length + lowData.cols() * lowData.rows(); Mat out = Mat.zeros(1, numCols, CV_32F).asMat(); FloatIndexer idx = out.createIndexer(); int j = 0; for (int i = 0; i < vhist.length; ++i, ++j) { idx.put(0, j, vhist[i]); } for (int i = 0; i < hhist.length; ++i, ++j) { idx.put(0, j, hhist[i]); } for (int x = 0; x < lowData.cols(); x++) { for (int y = 0; y < lowData.rows(); y++, ++j) { float val = lowData.ptr(x, y).get() & 0xFF; idx.put(0, j, val); } } return out; }
这段代码实现了提取图像的特征,通过计算垂直和水平的投影直方图以及图像的像素值来描述图像的特征。具体来说,该函数接受一个输入图像和一个指定的图像大小,如果需要将其缩放到指定的大小。然后,它计算输入图像的垂直和水平投影直方图,并将它们与图像像素值组合成一个特征向量。最后,它返回一个大小为1xN的矩阵,其中N是特征向量的长度。
阅读全文
相关推荐
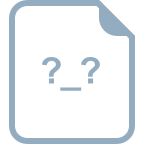
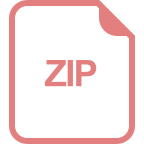
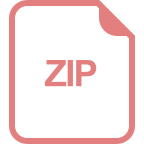
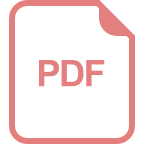
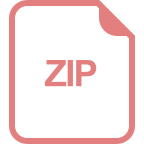
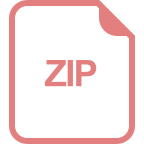
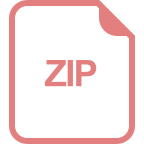
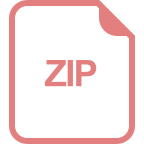
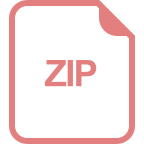
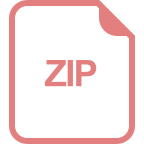
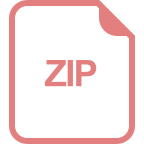
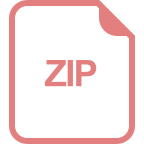
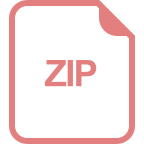
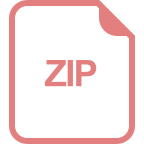
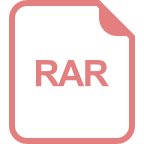
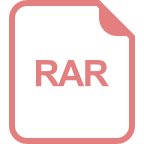
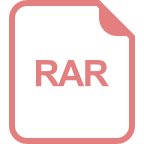
