编写一个linux程序,创建1个子进程,在子进程中列出/home/wanli/zs目录下以com开始、以tt结束的文件或子目录名,再等待3秒后终止子进程。父进程每秒钟检测子进程是否结束,若子进程未结束打印“The child process is not finished”,若子进程结束打印“The child process is finished”后结束。
时间: 2024-02-27 14:58:45 浏览: 69
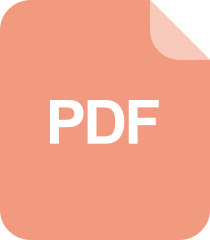
Linux 创建子进程执行任务的实现方法
好的,这是一个简单的 C 程序,实现了你的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <dirent.h>
#include <string.h>
int main() {
pid_t pid = fork();
if (pid == -1) { // fork 出错
perror("fork");
exit(EXIT_FAILURE);
}
if (pid == 0) { // 子进程
DIR *dir = opendir("/home/wanli/zs");
struct dirent *entry;
if (dir == NULL) {
perror("opendir");
exit(EXIT_FAILURE);
}
while ((entry = readdir(dir)) != NULL) {
if (strncmp(entry->d_name, "com", 3) == 0 && strcmp(entry->d_name + strlen(entry->d_name) - 2, "tt") == 0) {
printf("%s\n", entry->d_name);
}
}
closedir(dir);
sleep(3);
exit(EXIT_SUCCESS);
}
// 父进程
int status;
while (1) {
pid_t result = waitpid(pid, &status, WNOHANG);
if (result == -1) { // waitpid 出错
perror("waitpid");
exit(EXIT_FAILURE);
} else if (result == 0) { // 子进程未结束
printf("The child process is not finished\n");
sleep(1);
} else { // 子进程结束
printf("The child process is finished\n");
break;
}
}
return 0;
}
```
程序先通过 `fork()` 函数创建一个子进程,然后在子进程中打开 `/home/wanli/zs` 目录,遍历其中的所有文件和子目录,找到以 `com` 开头、以 `tt` 结尾的名称并输出。之后子进程通过 `sleep(3)` 函数等待 3 秒钟后退出。
父进程通过一个死循环不断调用 `waitpid()` 函数检测子进程是否已经退出。如果子进程未退出,父进程会打印出一条消息,并等待 1 秒钟后继续循环。当子进程退出后,父进程会打印出一条消息并结束程序。
阅读全文
相关推荐
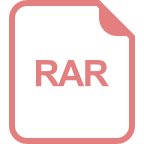

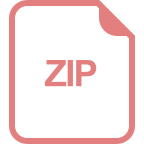
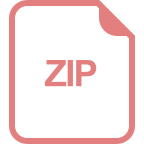
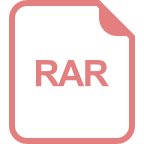
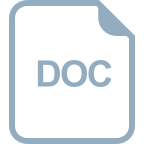
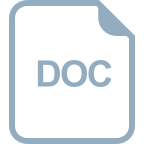
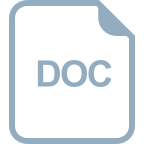
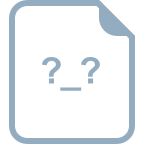
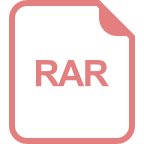
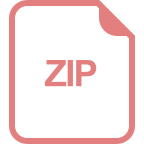
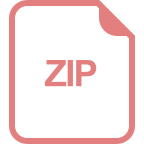
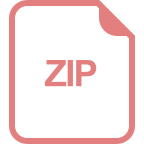
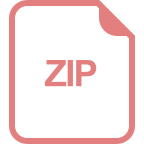