在Tensorflow环境框架下建立并训练BP神经网络模型,实现鸢尾花分类
时间: 2024-05-02 20:16:45 浏览: 9
好的,以下是在TensorFlow环境下建立并训练BP神经网络模型,实现鸢尾花分类的步骤:
1. 导入所需库
```python
import tensorflow as tf
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import OneHotEncoder
```
2. 加载数据集
```python
iris = load_iris()
x = iris.data
y = iris.target.reshape(-1, 1)
```
3. 进行数据预处理
```python
# 将类别标签进行one-hot编码
encoder = OneHotEncoder(sparse=False)
y = encoder.fit_transform(y)
# 将数据集划分为训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.2, random_state=1)
```
4. 定义模型的输入、输出和参数
```python
# 定义输入和输出占位符
x_input = tf.placeholder(shape=[None, 4], dtype=tf.float32)
y_output = tf.placeholder(shape=[None, 3], dtype=tf.float32)
# 定义模型参数
W = tf.Variable(tf.random_normal(shape=[4, 3]))
b = tf.Variable(tf.random_normal(shape=[3]))
```
5. 定义模型结构
```python
# 定义模型结构
model_output = tf.matmul(x_input, W) + b
```
6. 定义损失函数和优化器
```python
# 定义损失函数
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits_v2(logits=model_output, labels=y_output))
# 定义优化器
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01).minimize(loss)
```
7. 定义评估指标
```python
# 定义评估指标
correct_pred = tf.equal(tf.argmax(model_output, 1), tf.argmax(y_output, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
```
8. 训练模型
```python
# 定义训练参数
batch_size = 32
epochs = 100
# 创建会话并初始化变量
sess = tf.Session()
sess.run(tf.global_variables_initializer())
# 训练模型
for epoch in range(epochs):
for i in range(len(x_train) // batch_size):
batch_x = x_train[i*batch_size:(i+1)*batch_size]
batch_y = y_train[i*batch_size:(i+1)*batch_size]
sess.run(optimizer, feed_dict={x_input: batch_x, y_output: batch_y})
if (epoch+1) % 10 == 0:
acc = sess.run(accuracy, feed_dict={x_input: x_test, y_output: y_test})
print("Epoch: {}, Accuracy: {}".format(epoch+1, acc))
```
9. 测试模型
```python
# 计算测试集上的准确率
test_acc = sess.run(accuracy, feed_dict={x_input: x_test, y_output: y_test})
print("Test Accuracy: {}".format(test_acc))
```
完整代码如下:
```python
import tensorflow as tf
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import OneHotEncoder
# 加载数据集
iris = load_iris()
x = iris.data
y = iris.target.reshape(-1, 1)
# 将类别标签进行one-hot编码
encoder = OneHotEncoder(sparse=False)
y = encoder.fit_transform(y)
# 将数据集划分为训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.2, random_state=1)
# 定义输入和输出占位符
x_input = tf.placeholder(shape=[None, 4], dtype=tf.float32)
y_output = tf.placeholder(shape=[None, 3], dtype=tf.float32)
# 定义模型参数
W = tf.Variable(tf.random_normal(shape=[4, 3]))
b = tf.Variable(tf.random_normal(shape=[3]))
# 定义模型结构
model_output = tf.matmul(x_input, W) + b
# 定义损失函数
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits_v2(logits=model_output, labels=y_output))
# 定义优化器
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01).minimize(loss)
# 定义评估指标
correct_pred = tf.equal(tf.argmax(model_output, 1), tf.argmax(y_output, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
# 定义训练参数
batch_size = 32
epochs = 100
# 创建会话并初始化变量
sess = tf.Session()
sess.run(tf.global_variables_initializer())
# 训练模型
for epoch in range(epochs):
for i in range(len(x_train) // batch_size):
batch_x = x_train[i*batch_size:(i+1)*batch_size]
batch_y = y_train[i*batch_size:(i+1)*batch_size]
sess.run(optimizer, feed_dict={x_input: batch_x, y_output: batch_y})
if (epoch+1) % 10 == 0:
acc = sess.run(accuracy, feed_dict={x_input: x_test, y_output: y_test})
print("Epoch: {}, Accuracy: {}".format(epoch+1, acc))
# 计算测试集上的准确率
test_acc = sess.run(accuracy, feed_dict={x_input: x_test, y_output: y_test})
print("Test Accuracy: {}".format(test_acc))
```
相关推荐
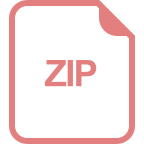
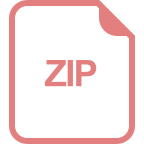
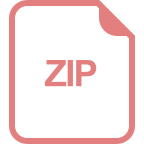














