c语言链表删除重复的结点
时间: 2024-02-25 11:52:42 浏览: 90
C 语言链表删除重复的结点可以分为两种情况:删除所有重复结点和仅保留一个重复结点。
删除所有重复结点的代码如下:
```c
struct ListNode {
int val;
struct ListNode* next;
};
struct ListNode* deleteDuplicates(struct ListNode* head) {
if (head == NULL) {
return NULL;
}
struct ListNode* dummy = (struct ListNode*)malloc(sizeof(struct ListNode));
dummy->next = head;
struct ListNode* pre = dummy;
struct ListNode* cur = head;
while (cur != NULL) {
while (cur->next != NULL && cur->val == cur->next->val) {
cur = cur->next;
}
if (pre->next == cur) {
pre = cur;
} else {
pre->next = cur->next;
}
cur = cur->next;
}
return dummy->next;
}
```
仅保留一个重复结点的代码如下:
```c
struct ListNode {
int val;
struct ListNode* next;
};
struct ListNode* deleteDuplicates(struct ListNode* head) {
if (head == NULL) {
return NULL;
}
struct ListNode* cur = head;
while (cur->next != NULL) {
if (cur->val == cur->next->val) {
struct ListNode* temp = cur->next;
cur->next = cur->next->next;
free(temp);
} else {
cur = cur->next;
}
}
return head;
}
```
这两段代码都是通过遍历链表,找到重复结点并删除。第一段代码会删除所有重复结点,第二段代码会仅保留一个重复结点。
阅读全文
相关推荐
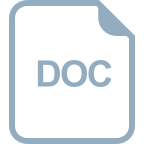



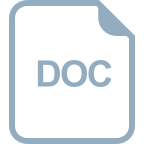
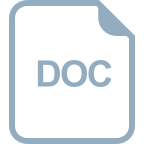
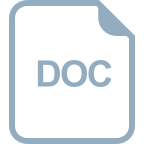
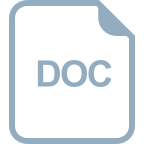






