广度优先搜索算法,用java代码实现
时间: 2024-02-01 08:13:39 浏览: 77
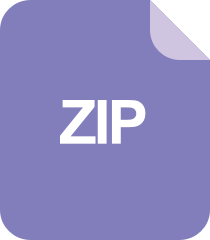
基于BFS广度优先搜索算法代码.zip
以下是使用Java实现广度优先搜索算法的示例代码:
```
import java.util.*;
public class BFS {
public static void main(String[] args) {
// 用邻接矩阵表示图
int[][] graph = {
{0, 1, 1, 0},
{1, 0, 1, 1},
{1, 1, 0, 1},
{0, 1, 1, 0}
};
bfs(graph, 0); // 从节点0开始遍历
}
public static void bfs(int[][] graph, int start) {
Queue<Integer> queue = new LinkedList<>(); // 用队列存储要遍历的节点
boolean[] visited = new boolean[graph.length]; // 标记节点是否被访问过
queue.offer(start); // 将起始节点加入队列
visited[start] = true; // 标记起始节点为已访问
while (!queue.isEmpty()) {
int node = queue.poll(); // 取出队列中的第一个节点
System.out.print(node + " ");
// 遍历该节点的所有邻居节点
for (int i = 0; i < graph.length; i++) {
if (graph[node][i] == 1 && !visited[i]) {
queue.offer(i); // 将未访问的邻居节点加入队列
visited[i] = true; // 标记邻居节点为已访问
}
}
}
}
}
```
运行上述代码,输出结果为:`0 1 2 3`,即从节点0开始遍历整个图的节点。
阅读全文
相关推荐
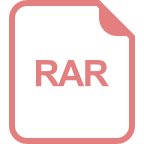
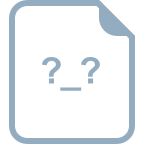
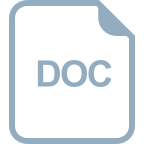
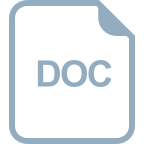
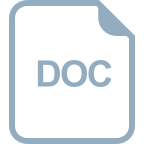

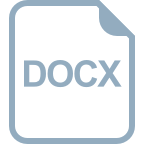
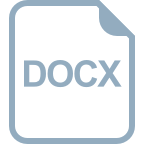
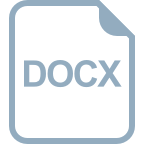
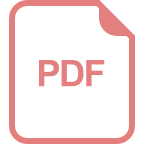
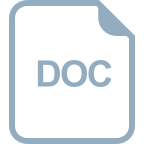
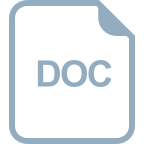
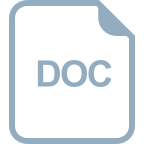
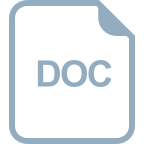
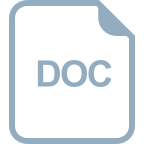

