使用多线程批量新增海量数据 使用Java以及 mubatis postgresql
时间: 2024-01-11 14:03:16 浏览: 105
使用Java和MyBatis操作PostgreSQL数据库可以实现多线程批量新增海量数据的操作。具体步骤如下:
1. 创建数据源:使用Java的JDBC API连接PostgreSQL数据库,创建数据源。
2. 配置MyBatis:使用MyBatis的配置文件配置数据源和Mapper。
3. 编写Mapper接口:编写Mapper接口,定义新增数据的方法。
4. 编写多线程代码:根据数据量大小和硬件资源情况,确定线程数量和每个线程处理数据的数量。在每个线程中,使用MyBatis的SqlSession执行新增数据的方法。
5. 处理结果合并:每个线程执行完后,将新增数据的结果合并到一个集合中。
6. 事务控制:在整个过程中,需要使用事务控制来保证数据的完整性和正确性。在每个线程中,使用MyBatis的SqlSession开启事务,执行完毕后提交事务。
下面是一个简单的Java多线程批量新增数据的示例代码:
```java
public class MultiThreadInsert {
private static final int THREAD_NUM = 10; // 线程数量
private static final int BATCH_SIZE = 1000; // 每个线程处理数据的数量
public static void main(String[] args) throws Exception {
// 创建数据源
DataSource dataSource = createDataSource();
// 配置MyBatis
SqlSessionFactory sqlSessionFactory = createSqlSessionFactory(dataSource);
// 获取Mapper
MyMapper mapper = sqlSessionFactory.openSession().getMapper(MyMapper.class);
// 创建线程池
ExecutorService executorService = Executors.newFixedThreadPool(THREAD_NUM);
// 创建结果集
List<Integer> resultList = Collections.synchronizedList(new ArrayList<>());
// 创建CountDownLatch
CountDownLatch countDownLatch = new CountDownLatch(THREAD_NUM);
// 创建线程
for (int i = 0; i < THREAD_NUM; i++) {
executorService.execute(new InsertTask(mapper, BATCH_SIZE, resultList, countDownLatch));
}
// 等待所有线程执行完毕
countDownLatch.await();
// 关闭线程池和数据源
executorService.shutdown();
dataSource.getConnection().close();
// 输出结果
System.out.println("Total insert count: " + resultList.stream().mapToInt(Integer::intValue).sum());
}
private static DataSource createDataSource() {
// 创建PostgreSQL数据源
PGSimpleDataSource dataSource = new PGSimpleDataSource();
dataSource.setServerName("localhost");
dataSource.setDatabaseName("test");
dataSource.setUser("postgres");
dataSource.setPassword("postgres");
return dataSource;
}
private static SqlSessionFactory createSqlSessionFactory(DataSource dataSource) throws Exception {
// 创建MyBatis配置
Configuration configuration = new Configuration();
configuration.addMapper(MyMapper.class);
// 创建MyBatis SqlSessionFactory
SqlSessionFactoryBuilder builder = new SqlSessionFactoryBuilder();
return builder.build(configuration, dataSource);
}
private static class InsertTask implements Runnable {
private final MyMapper mapper;
private final int batchSize;
private final List<Integer> resultList;
private final CountDownLatch countDownLatch;
public InsertTask(MyMapper mapper, int batchSize, List<Integer> resultList, CountDownLatch countDownLatch) {
this.mapper = mapper;
this.batchSize = batchSize;
this.resultList = resultList;
this.countDownLatch = countDownLatch;
}
@Override
public void run() {
try {
// 开启事务
SqlSession sqlSession = mapper.getSqlSession();
sqlSession.getConnection().setAutoCommit(false);
MyMapper batchMapper = sqlSession.getMapper(MyMapper.class);
int count = 0;
// 批量新增数据
for (int i = 0; i < batchSize; i++) {
int insertCount = batchMapper.insertData(new Data());
count += insertCount;
}
// 提交事务
sqlSession.commit();
// 记录新增数据的数量
resultList.add(count);
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭SqlSession
mapper.getSqlSession().close();
// 计数器减1
countDownLatch.countDown();
}
}
}
}
```
其中,MyMapper是Mapper接口,定义了新增数据的方法:
```java
public interface MyMapper {
int insertData(Data data);
}
```
Data是数据实体类,对应要新增的数据。以上代码仅供参考,实际应用中需要根据具体情况进行调整和优化。
阅读全文
相关推荐
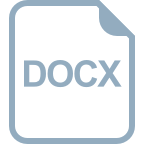
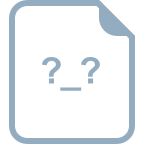
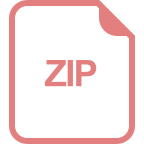
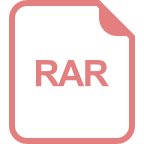
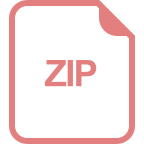
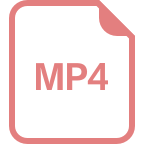
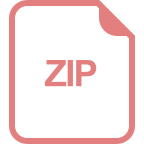
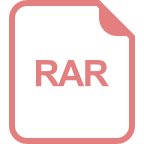
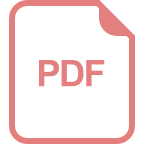