如何模拟出真实环境下的噪声干扰,使用python代码
时间: 2023-12-03 14:46:27 浏览: 60
模拟真实环境下的噪声干扰,可以使用Python中的一些信号处理库。比如,可以使用`numpy`和`scipy`库来生成不同类型的噪声信号。
下面是一些示例代码:
1. 添加高斯白噪声
```python
import numpy as np
# 生成长度为1000的高斯白噪声信号
noise = np.random.normal(0, 1, 1000)
# 将高斯白噪声信号添加到原始信号上
signal_with_noise = signal + noise
```
2. 添加均匀分布噪声
```python
import numpy as np
# 生成长度为1000的均匀分布噪声信号
noise = np.random.uniform(-1, 1, 1000)
# 将均匀分布噪声信号添加到原始信号上
signal_with_noise = signal + noise
```
3. 添加正弦噪声
```python
import numpy as np
# 生成长度为1000的正弦波信号
t = np.linspace(0, 1, 1000)
signal = np.sin(2 * np.pi * 10 * t)
# 生成长度为1000的正弦噪声信号
noise = np.sin(2 * np.pi * 50 * t)
# 将正弦噪声信号添加到原始信号上
signal_with_noise = signal + noise
```
4. 添加高斯滤波器噪声
```python
import numpy as np
from scipy.signal import butter, lfilter
# 生成长度为1000的正弦波信号
t = np.linspace(0, 1, 1000)
signal = np.sin(2 * np.pi * 10 * t)
# 定义一个高斯滤波器
def butter_bandpass_filter(data, lowcut, highcut, fs, order=5):
nyq = 0.5 * fs
low = lowcut / nyq
high = highcut / nyq
b, a = butter(order, [low, high], btype='band')
y = lfilter(b, a, data)
return y
# 将原始信号通过高斯滤波器
fs = 1000 # 采样率
lowcut = 50 # 最低频率
highcut = 200 # 最高频率
filtered_signal = butter_bandpass_filter(signal, lowcut, highcut, fs, order=5)
# 生成长度为1000的高斯白噪声信号
noise = np.random.normal(0, 1, 1000)
# 将高斯白噪声信号添加到滤波后的信号上
signal_with_noise = filtered_signal + noise
```
以上代码示例可以根据需要进行调整和改进,以模拟出更加真实的噪声干扰。
相关推荐
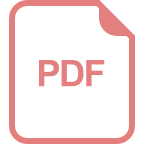
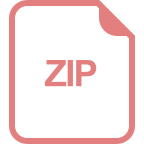
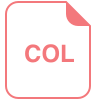
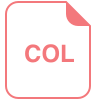












