扮演一位python研发人员,优化这段代码,识别出图片位置时划绿色线标识出图片坐标
时间: 2024-03-02 07:48:13 浏览: 99
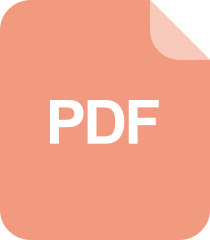
python找出在原图中的位置-python提取照片坐标信息的实例代码
好的,我会尝试对这段代码进行优化,在识别出图片位置时,使用 OpenCV 库来划绿色线标识出图片坐标。
首先,需要安装 OpenCV 库,可以使用以下命令:
```python
pip install opencv-python
```
然后,对代码进行修改,添加以下代码:
```python
import cv2
def find_image_location(image_path):
# 加载图片
img = cv2.imread(image_path)
# 查找图片位置
location = pyautogui.locateOnScreen(image_path)
if location is not None:
# 获取图片中心点的坐标
x, y = pyautogui.center(location)
# 在图片上标识出位置坐标
cv2.line(img, (x-10, y), (x+10, y), (0, 255, 0), 2)
cv2.line(img, (x, y-10), (x, y+10), (0, 255, 0), 2)
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
return (x, y)
else:
return None
```
这段代码使用 OpenCV 库中的 `cv2.imread` 函数加载图片,然后在图片上使用 `cv2.line` 函数划绿色线标识出图片坐标。
最后,完整的代码如下:
```python
import tkinter as tk
from tkinter import filedialog
import pyautogui
import cv2
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.select_button = tk.Button(self)
self.select_button["text"] = "选择图片"
self.select_button["command"] = self.select_image
self.select_button.pack(side="top")
self.result_label = tk.Label(self)
self.result_label.pack(side="bottom")
def select_image(self):
# 弹出文件选择对话框选择要查找的图片
file_path = filedialog.askopenfilename(title="选择图片文件", filetypes=[("Image files", "*.png;*.jpg;*.jpeg")])
# 如果选择了图片则查找图片位置
if file_path:
location = find_image_location(file_path)
if location is not None:
self.result_label["text"] = f"图片 {file_path} 的位置坐标为:{location}"
else:
self.result_label["text"] = f"图片 {file_path} 不存在于桌面"
def find_image_location(image_path):
# 加载图片
img = cv2.imread(image_path)
# 查找图片位置
location = pyautogui.locateOnScreen(image_path)
if location is not None:
# 获取图片中心点的坐标
x, y = pyautogui.center(location)
# 在图片上标识出位置坐标
cv2.line(img, (x-10, y), (x+10, y), (0, 255, 0), 2)
cv2.line(img, (x, y-10), (x, y+10), (0, 255, 0), 2)
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
return (x, y)
else:
return None
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
这样,当用户选择一个图片后,程序会在屏幕上查找图片位置,并在图片上标识出位置坐标。
阅读全文
相关推荐
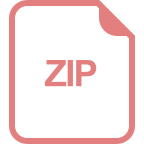
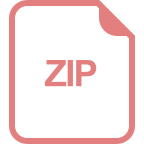
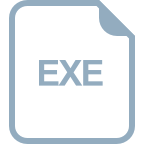
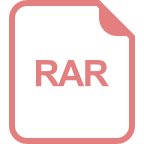
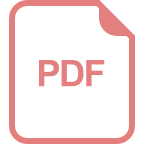
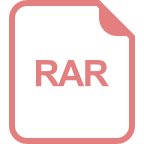
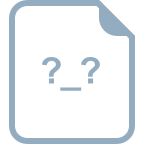
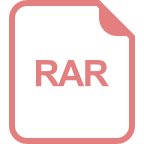
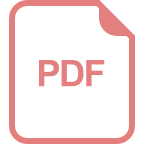
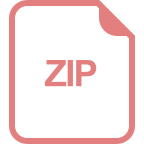
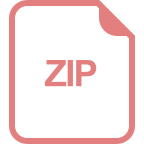
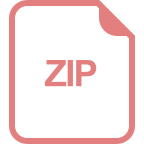
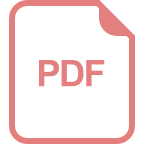