Java编写程序,要求如下自行编码产生常见异常。 main方法 事先定义好一个大小为5的数组。 根据屏幕输入产生相应异常。 提示:可以使用System.out.println(e)打印异常对象的信息,其中e为捕获到的异常对象。 输入说明: arr 代表产生访问数组是产生的异常。然后输入下标,如果抛出ArrayIndexOutOfBoundsException异常则显示,如果不抛出异常则不显示。 null,产生NullPointerException cast,尝试将String对象强制转化为Integ
时间: 2023-12-03 21:44:47 浏览: 176
er类型,如果抛出ClassCastException异常则显示,如果不抛出异常则不显示。 div,输入两个整数,尝试进行除法运算,如果抛出ArithmeticException异常则显示,如果不抛出异常则不显示。 io,尝试读取一个不存在的文件,如果抛出FileNotFoundException异常则显示,如果不抛出异常则不显示。
以下是Java代码实现:
```java
import java.util.Scanner;
import java.io.File;
import java.io.FileNotFoundException;
public class ExceptionDemo {
public static void main(String[] args) {
int[] arr = new int[5];
Scanner input = new Scanner(System.in);
String choice = "";
do {
System.out.println("请选择要产生的异常类型:");
System.out.println("arr:访问数组产生异常");
System.out.println("null:空指针异常");
System.out.println("cast:类型转换异常");
System.out.println("div:除以0异常");
System.out.println("io:文件读取异常");
System.out.println("exit:退出程序");
choice = input.next();
try {
if (choice.equals("arr")) {
System.out.print("请输入数组下标:");
int index = input.nextInt();
arr[index] = 1;
} else if (choice.equals("null")) {
String str = null;
System.out.println(str.length());
} else if (choice.equals("cast")) {
String str = "123";
Integer num = (Integer) str;
} else if (choice.equals("div")) {
System.out.print("请输入被除数:");
int dividend = input.nextInt();
System.out.print("请输入除数:");
int divisor = input.nextInt();
int result = dividend / divisor;
System.out.println("结果为:" + result);
} else if (choice.equals("io")) {
File file = new File("nonexistent.txt");
Scanner fileInput = new Scanner(file);
} else if (!choice.equals("exit")) {
System.out.println("无效的选项,请重新选择。");
}
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("产生了数组越界异常:" + e);
} catch (NullPointerException e) {
System.out.println("产生了空指针异常:" + e);
} catch (ClassCastException e) {
System.out.println("产生了类型转换异常:" + e);
} catch (ArithmeticException e) {
System.out.println("产生了除以0异常:" + e);
} catch (FileNotFoundException e) {
System.out.println("产生了文件读取异常:" + e);
}
} while (!choice.equals("exit"));
}
}
```
运行程序后,根据提示选择要产生的异常类型并输入相应的参数,程序会捕获并处理相应的异常,并打印异常信息。
阅读全文
相关推荐
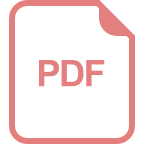
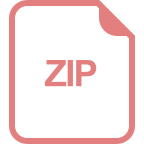
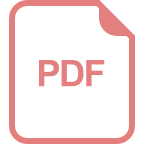
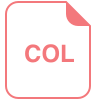
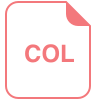
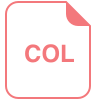
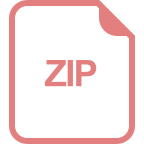
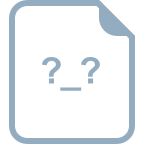
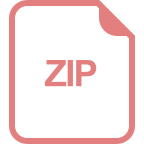
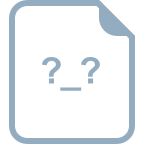
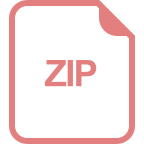
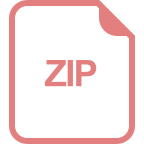