使用Scala 实现 目录及文件copy操作
时间: 2024-02-04 13:04:30 浏览: 31
以下是使用 Scala 实现目录及文件 copy 操作的示例代码:
```scala
import java.io._
object FileCopy {
def main(args: Array[String]): Unit = {
val source = new File("path/to/source/directory")
val destination = new File("path/to/destination/directory")
copyDirectory(source, destination)
}
def copyDirectory(source: File, destination: File): Unit = {
if (source.isDirectory) {
if (!destination.exists()) {
destination.mkdir()
}
val files = source.listFiles()
for (f <- files) {
val newFile = new File(destination.getAbsolutePath + File.separator + f.getName)
copyDirectory(f, newFile)
}
} else {
val in = new FileInputStream(source)
val out = new FileOutputStream(destination)
val buffer = new Array[Byte](1024)
var length = in.read(buffer)
while (length > 0) {
out.write(buffer, 0, length)
length = in.read(buffer)
}
in.close()
out.close()
}
}
}
```
在上述代码中,我们首先定义了源目录和目标目录,然后调用 `copyDirectory` 方法来执行目录和文件的复制操作。如果源目录是一个目录,我们会检查目标目录是否存在,如果不存在则创建它,并且遍历源目录中的所有文件和子目录。对于每个文件,我们都会创建一个新的文件对象,并递归调用 `copyDirectory` 方法来复制文件到目标目录中。如果源目录是一个文件,我们会使用 `FileInputStream` 和 `FileOutputStream` 来读取和写入文件内容,并使用一个缓冲区来提高性能。最后,我们关闭输入和输出流并退出方法。
相关推荐
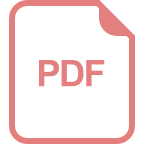
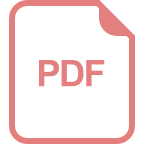
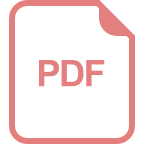














