js生成随机完全平方公式题目
时间: 2023-09-06 07:11:32 浏览: 86
好的,以下是一种生成随机完全平方公式题目的方法:
```javascript
function generateQuestion() {
const a = Math.floor(Math.random() * 10) + 1;
const b = Math.floor(Math.random() * 10) + 1;
const c = a * b;
return `求解 ${a}x² + ${2 * b}x + ${b * b} = ${c}`;
}
```
这个函数会生成一个类似于 "求解 3x² + 10x + 9 = 27" 的题目,其中 x 为未知数,a、b、c 都是随机生成的正整数。该题目的解为 x = 3。
相关问题
js随机完全平方公式计算题
如果你想在 JS 中生成一个随机的完全平方数,可以使用以下公式:
```javascript
var randomSquare = Math.pow(Math.floor(Math.sqrt(Math.random() * 100)), 2);
```
这个公式的作用是:
1. `Math.random()` 生成一个 0 到 1 之间的随机数。
2. `Math.sqrt()` 对这个随机数进行平方根运算,得到一个 0 到 1 之间的数。
3. `Math.floor()` 对这个数向下取整,得到一个 0 到 0.9999... 之间的整数。
4. `Math.pow()` 对这个整数进行平方运算,得到一个完全平方数。
上述公式中的 `100` 可以替换为你想要的任何数,它决定了生成的随机数的范围。
自动生成随机题目代码js
在JavaScript中生成随机题目代码通常涉及到生成随机数、字符串拼接以及条件判断等操作。下面是一个简单的示例,展示如何创建一个随机选择运算符并生成加减乘除的数学题:
```javascript
function generateRandomMathQuestion() {
const operators = ['+', '-', '*', '/'];
const num1 = Math.floor(Math.random() * 10) + 1; // 生成1到10之间的整数
const num2 = Math.floor(Math.random() * 10) + 1;
let question = `${num1} ` + operators[Math.floor(Math.random() * operators.length)]; // 随机选取一个运算符
// 如果运算符是除法,需要处理除数为零的情况
if (operators.includes('/')) {
while (num2 === 0) { // 如果随机出的是除法,且第二个数字为零,则重试
num2 = Math.floor(Math.random() * 10) + 1;
}
}
question += ` ${num2}`;
return question;
}
// 调用函数生成一个随机题目
console.log(generateRandomMathQuestion());
```
阅读全文
相关推荐
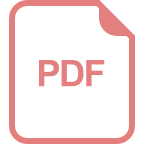
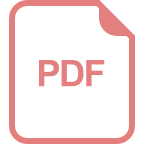
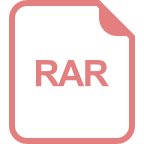

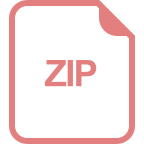
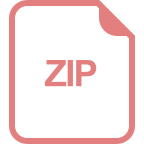
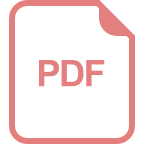
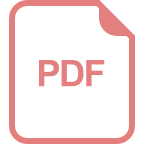
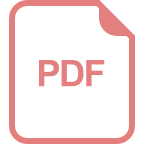
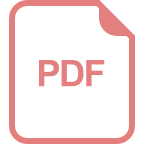
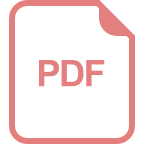
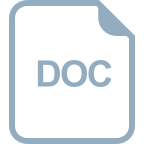

