java web3j调用合约调用之后,怎么知道操作是否成功
时间: 2023-11-22 08:04:32 浏览: 37
调用合约后,Web3j会返回一个TransactionReceipt对象,该对象包含了交易的详细信息,包括交易的状态。通过检查TransactionReceipt对象的getStatus()方法,可以知道交易的状态。如果状态为"0x1",则表示操作成功;如果状态为"0x0",则表示操作失败。下面是一个调用合约并检查交易状态的示例代码:
```
TransactionReceipt receipt = contract.transfer(new Address("0x123..."), new Uint256(100)).send();
if (receipt.getStatus().equals("0x1")) {
System.out.println("Transfer succeeded!");
} else {
System.out.println("Transfer failed!");
}
```
相关问题
智能合约 web3j Java_Java使用web3j调用智能合约
要使用 Java 调用智能合约,需要使用 web3j 库。web3j 是一个用于与以太坊网络进行交互的轻量级 Java 库。下面是使用 web3j 调用智能合约的一些基本步骤:
1.在项目中添加 web3j 库的依赖:
```
<dependency>
<groupId>org.web3j</groupId>
<artifactId>core</artifactId>
<version>4.5.14</version>
</dependency>
<dependency>
<groupId>org.web3j</groupId>
<artifactId>crypto</artifactId>
<version>4.5.14</version>
</dependency>
<dependency>
<groupId>org.web3j</groupId>
<artifactId>abi</artifactId>
<version>4.5.14</version>
</dependency>
```
2.创建一个与以太坊节点进行连接的 Web3j 对象:
```
Web3j web3j = Web3j.build(new HttpService("http://localhost:8545"));
```
3.构建一个与智能合约进行交互的 Credentials 对象:
```
Credentials credentials = WalletUtils.loadCredentials("password", "/path/to/walletfile");
```
4.使用智能合约的地址和 ABI 构建一个 Contract 对象:
```
String contractAddress = "0x123456...";
String contractABI = "...";
Contract contract = Contract.load(contractAddress, web3j, credentials, new DefaultGasProvider(), contractABI);
```
5.调用智能合约的方法:
```
TransactionReceipt transactionReceipt = contract.methodName(param1, param2).send();
```
其中,methodName 是智能合约中定义的方法名,param1 和 param2 是该方法的参数。send() 方法用于发送交易并等待交易被打包,并返回交易回执。
以上是使用 web3j 调用智能合约的基本步骤,具体的实现需要根据实际情况进行调整和优化。
java 调用合约方法的
方式有两种:一种是通过 web3j 框架调用;另一种是通过 ethereumj 框架调用。
1. web3j 框架调用合约方法
web3j 是一个基于 Java 的以太坊客户端库,提供了访问以太坊网络的 API。可以使用 web3j 框架调用合约方法,具体步骤如下:
1)创建 web3j 客户端对象
Web3j web3j = Web3j.build(new HttpService("http://localhost:8545"));
2)加载合约 ABI 文件
String contractAddress = "0x123456...";
String contractABI = "合约ABI文件内容";
Contract contract = Contract.load(contractAddress, web3j, credentials, gasPrice, gasLimit);
3)调用合约方法
TransactionReceipt receipt = contract.someMethod().send();
其中 someMethod() 是合约的一个方法,可以根据合约的具体情况调用不同的方法。
2. ethereumj 框架调用合约方法
ethereumj 是一个 Java 实现的以太坊客户端,可以使用 ethereumj 框架调用合约方法,具体步骤如下:
1)创建 ethereumj 客户端对象
ECKey key = ECKey.fromPrivate(privateKey);
InMemoryStorageProvider storageProvider = new InMemoryStorageProvider();
Repository repository = new Repository(storageProvider);
BlockchainImpl blockchain = new BlockchainImpl(repository);
EthereumImpl ethereum = new EthereumImpl(blockchain);
ethereum.addListener(new EthereumListenerAdapter());
ethereum.connect();
2)加载合约 ABI 文件
String contractAddress = "0x123456...";
String contractABI = "合约ABI文件内容";
Contract contract = new Contract(new Address(contractAddress), ethereum, key, contractABI.getBytes());
3)调用合约方法
TransactionReceipt receipt = contract.callFunction("someMethod", args);
其中 someMethod 是合约的一个方法名,args 是方法的参数。
相关推荐
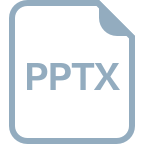
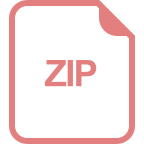
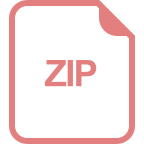
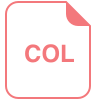
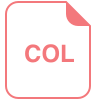







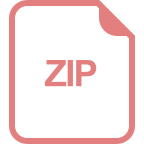
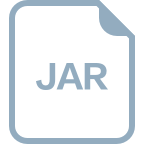
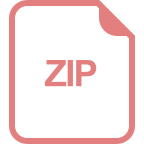
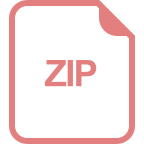