python用pyqt编写一个应用界面
时间: 2023-07-18 09:10:15 浏览: 47
首先,你需要安装 PyQt 库,安装命令为:
```python
pip install PyQt5
```
然后,在 Python 中创建一个新的文件,比如命名为 main.py。在文件中导入 PyQt5 库和必要的模块:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton
```
然后,创建一个窗口并设置窗口的标题和大小:
```python
class App(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('My PyQt App')
self.setGeometry(100, 100, 400, 300)
# 以下为添加控件的代码
```
接下来,你可以添加窗口中的控件,比如标签和按钮:
```python
class App(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('My PyQt App')
self.setGeometry(100, 100, 400, 300)
# 添加标签
lbl = QLabel('Hello World!', self)
lbl.move(150, 50)
# 添加按钮
btn = QPushButton('Quit', self)
btn.setToolTip('Click to quit the application')
btn.move(150, 200)
btn.clicked.connect(self.close)
```
最后,在主程序中启动应用程序:
```python
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
ex.show()
sys.exit(app.exec_())
```
这样,一个简单的 PyQt 应用程序就完成了。你可以运行它并查看效果。
相关推荐
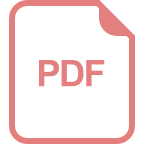
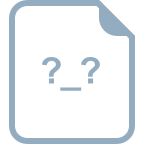
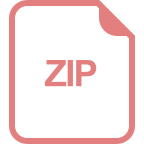














