请详细讲解qml的listview如何和QAbstractListModel配合使用,举例如何把qt读取到的多个文件数据(含有QString,QImage)发送到qml的listview中显示,请给出完整详细的代码例子
时间: 2024-05-01 16:17:07 浏览: 158
QML的ListView是用来展示列表数据的控件,而QAbstractListModel是Qt中用来实现自定义数据模型的类。通过将二者配合使用,我们可以实现将自定义数据以列表的形式展示在QML界面中。
下面是一个简单的例子,演示如何将Qt读取到的多个文件数据(含有QString和QImage)发送到QML的ListView中显示。
1. 创建自定义数据模型
首先,我们需要创建一个继承自QAbstractListModel的自定义数据模型。这个数据模型需要实现以下几个方法:
- rowCount():返回数据模型中的行数;
- data():返回指定行和角色的数据;
- roleNames():返回数据模型中所有角色的名称。
下面是一个示例的自定义数据模型,其中包含了一个QString类型和一个QImage类型的数据:
```cpp
class FileListModel : public QAbstractListModel
{
Q_OBJECT
public:
enum FileRoles {
FileNameRole = Qt::UserRole + 1,
ImageRole
};
explicit FileListModel(QObject *parent = nullptr);
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
QHash<int, QByteArray> roleNames() const override;
void addFile(const QString& fileName);
private:
struct FileData {
QString fileName;
QImage image;
};
QList<FileData> m_files;
};
```
2. 实现自定义数据模型的方法
接下来,我们需要实现自定义数据模型中的方法。在这个例子中,我们实现了rowCount()、data()和roleNames()方法,其中roleNames()方法返回了两个角色的名称:FileNameRole和ImageRole。
```cpp
FileListModel::FileListModel(QObject *parent)
: QAbstractListModel(parent)
{
}
int FileListModel::rowCount(const QModelIndex &parent) const
{
if (parent.isValid())
return 0;
return m_files.count();
}
QVariant FileListModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid())
return QVariant();
const FileData& fileData = m_files[index.row()];
switch (role) {
case FileNameRole:
return fileData.fileName;
case ImageRole:
return QVariant::fromValue(fileData.image);
default:
return QVariant();
}
}
QHash<int, QByteArray> FileListModel::roleNames() const
{
QHash<int, QByteArray> roles;
roles[FileNameRole] = "fileName";
roles[ImageRole] = "image";
return roles;
}
```
3. 在QML中使用ListView展示数据
接下来,我们需要在QML中使用ListView来展示自定义数据模型中的数据。为了实现这个功能,我们需要将ListView的model属性设置为我们创建的自定义数据模型的实例。
下面是一个简单的QML界面,其中包含了一个ListView和一个Delegate,用来展示自定义数据模型中的数据:
```qml
import QtQuick 2.0
ListView {
id: fileView
anchors.fill: parent
model: fileModel
delegate: Rectangle {
width: 200; height: 200
color: "white"
border.width: 2
border.color: "black"
Image {
source: image
anchors.fill: parent
fillMode: Image.PreserveAspectFit
}
Text {
text: fileName
font.bold: true
font.pixelSize: 12
anchors.bottom: parent.bottom
anchors.horizontalCenter: parent.horizontalCenter
padding: 5
}
}
}
```
4. 将数据添加到自定义数据模型中
最后,我们需要将Qt读取到的多个文件数据添加到自定义数据模型中。下面是一个简单的示例代码,其中通过addFile()方法将文件名和图像添加到自定义数据模型中:
```cpp
FileListModel fileModel;
for (const QString& fileName : fileNames) {
QImage image(fileName);
if (image.isNull())
continue;
fileModel.addFile(fileName);
}
QQmlApplicationEngine engine;
engine.rootContext()->setContextProperty("fileModel", &fileModel);
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (engine.rootObjects().isEmpty())
return -1;
```
完整的代码如下:
阅读全文
相关推荐





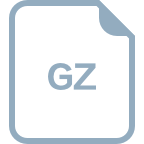











