mtx_lock 的实现源码
时间: 2023-11-28 11:04:15 浏览: 38
mtx_lock 是 POSIX 标准中的一个互斥锁函数,它的作用是用来保证多线程访问共享资源时的线程同步。
在 Linux 系统中,mtx_lock 函数的实现源码主要在 pthread_mutex_lock 函数中。以下是 pthread_mutex_lock 函数的简化版本:
```c
int pthread_mutex_lock(pthread_mutex_t *mutex) {
// 省略参数检查
// 尝试获取锁
if (__pthread_mutex_trylock_internal(mutex) == 0)
return 0;
// 获取锁失败,需要阻塞等待
return __pthread_mutex_lock_full(mutex, NULL);
}
```
上面的代码中,__pthread_mutex_trylock_internal 函数是用来尝试获取互斥锁的,如果获取成功,直接返回 0;如果获取失败,则调用 __pthread_mutex_lock_full 函数进行阻塞等待。
__pthread_mutex_lock_full 函数的实现比较复杂,其中涉及到了条件变量、信号量等多种线程同步原语。简化版本的代码如下:
```c
int __pthread_mutex_lock_full(pthread_mutex_t *mutex, const struct timespec *abstime) {
// 省略参数检查
// 加入等待队列
__pthread_mutex_add_to_pending_lock(mutex);
// 循环等待锁
while (1) {
// 尝试获取锁
if (__pthread_mutex_trylock_internal(mutex) == 0) {
// 从等待队列中删除
__pthread_mutex_remove_from_pending_lock(mutex);
return 0;
}
// 如果设置了超时时间,则等待一段时间后返回
if (abstime != NULL) {
if (__pthread_mutex_timedwait(mutex, abstime) != 0) {
__pthread_mutex_remove_from_pending_lock(mutex);
return ETIMEDOUT;
}
} else {
// 阻塞等待
__pthread_mutex_wait(mutex);
}
}
}
```
上面的代码中,__pthread_mutex_add_to_pending_lock 函数是用来把当前线程加入到等待队列中的;__pthread_mutex_remove_from_pending_lock 函数是用来把当前线程从等待队列中删除的;__pthread_mutex_timedwait 函数是带有超时时间的等待函数,如果等待时间超过了指定的时间,则返回 ETIMEDOUT 错误码;__pthread_mutex_wait 函数是无限期等待的函数,直到获取到锁为止。
综上所述,pthread_mutex_lock 函数的实现源码比较复杂,其中涉及到了多种线程同步原语,但是其基本思路可以概括为:加入等待队列 -> 尝试获取锁 -> 获取锁成功或者等待锁释放。
相关推荐
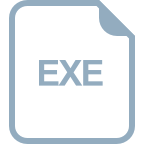














