实现链式存储的泛型队列
时间: 2024-03-18 22:15:39 浏览: 34
以下是使用链式存储实现泛型队列的示例代码:
```java
public class Queue<T> {
private Node<T> head;
private Node<T> tail;
private int size;
private static class Node<T> {
private final T value;
private Node<T> next;
public Node(T value) {
this.value = value;
}
}
public Queue() {
this.head = null;
this.tail = null;
this.size = 0;
}
public void enqueue(T value) {
Node<T> newNode = new Node<>(value);
if (isEmpty()) {
head = newNode;
tail = newNode;
} else {
tail.next = newNode;
tail = newNode;
}
size++;
}
public T dequeue() {
if (isEmpty()) {
throw new NoSuchElementException("Queue is empty");
}
T value = head.value;
head = head.next;
size--;
if (isEmpty()) {
tail = null;
}
return value;
}
public T peek() {
if (isEmpty()) {
throw new NoSuchElementException("Queue is empty");
}
return head.value;
}
public boolean isEmpty() {
return size == 0;
}
public int size() {
return size;
}
}
```
在这个实现中,我们使用了一个内部类 Node 来表示队列中的节点。每个节点包含一个值和一个指向下一个节点的指针。我们使用头指针 head 和尾指针 tail 来跟踪队列的起始和结束位置,并使用 size 变量来记录队列的大小。
enqueue() 方法将一个新节点添加到队列的末尾。如果队列为空,则同时更新头指针和尾指针。否则,只需要将新节点链接到当前尾节点的 next 指针,并将尾指针更新为新节点。
dequeue() 方法从队列的开头删除一个节点,并返回其值。如果队列为空,则抛出 NoSuchElementException 异常。否则,我们更新头指针,将队列大小减少,并在队列为空时更新尾指针。
peek() 方法返回队列的开头节点的值,但不删除它。如果队列为空,则抛出 NoSuchElementException 异常。
isEmpty() 方法检查队列是否为空。
size() 方法返回队列的大小。
这个实现是线程不安全的,因为它没有考虑并发访问。如果您需要在多个线程中使用此队列,请考虑添加同步或使用线程安全的队列实现。
相关推荐
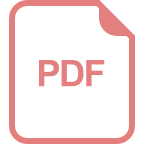
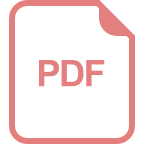














