acc可视化界面做些什么
时间: 2023-10-31 13:04:47 浏览: 23
ACC(自适应巡航控制系统)可视化界面主要用于显示和设置ACC系统的工作状态和参数,包括以下功能:
1. 显示当前车速和距离前车的距离等基本信息;
2. 显示ACC系统的工作状态,例如开启状态、暂停状态、跟车状态等;
3. 允许用户设置ACC的工作模式,例如常规模式、运动模式、经济模式等;
4. 允许用户设置ACC的跟车距离,例如近距离、中距离、远距离等;
5. 允许用户设置ACC的最高速度和最低速度限制;
6. 显示ACC系统的警告和提示信息,例如跟车距离过近、前方车辆变道等;
7. 允许用户手动控制车速和跟车距离,以应对特殊情况。
通过ACC可视化界面,司机可以更加直观地了解ACC系统的工作状态和参数,更加安全地驾驶车辆。
相关问题
acc使用的可视化界面工具
ACC(AutoML Component Configurator)可视化界面工具是一款基于Python语言和Jupyter Notebook的开源工具,主要用于自动机器学习(AutoML)任务的可视化配置和管理。它提供了一系列可视化的交互式界面,帮助用户更加便捷地进行机器学习任务的配置和管理,包括数据集的导入、特征工程的配置、模型的选择和调优,以及模型训练和预测等。同时,ACC还支持多种机器学习框架和算法,如Scikit-learn、XGBoost、LightGBM、Keras等,可以满足不同应用场景的需求。
基于TensorFlow的CNN实现Mnist手写数字识别,带有可视化界面
好的,以下是基于TensorFlow的CNN实现Mnist手写数字识别的代码,带有可视化界面。
首先需要安装必要的库,包括TensorFlow、Tkinter和Pillow:
```
pip install tensorflow
pip install tkinter
pip install pillow
```
然后,我们需要下载Mnist数据集。可以在TensorFlow的官方GitHub页面找到下载链接,或者使用以下代码下载:
```
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
```
接下来,我们可以开始构建CNN模型。下面的代码展示了一个简单的CNN模型:
```
import tensorflow as tf
# Define parameters
learning_rate = 0.001
training_iters = 20000
batch_size = 128
display_step = 10
# Network parameters
n_input = 784
n_classes = 10
dropout = 0.75
# Create placeholders
x = tf.placeholder(tf.float32, [None, n_input])
y = tf.placeholder(tf.float32, [None, n_classes])
keep_prob = tf.placeholder(tf.float32)
# Create convnet
def conv2d(x, W, b, strides=1):
x = tf.nn.conv2d(x, W, strides=[1, strides, strides, 1], padding='SAME')
x = tf.nn.bias_add(x, b)
return tf.nn.relu(x)
def maxpool2d(x, k=2):
return tf.nn.max_pool(x, ksize=[1, k, k, 1], strides=[1, k, k, 1], padding='SAME')
def conv_net(x, weights, biases, dropout):
x = tf.reshape(x, shape=[-1, 28, 28, 1])
conv1 = conv2d(x, weights['wc1'], biases['bc1'])
conv1 = maxpool2d(conv1, k=2)
conv2 = conv2d(conv1, weights['wc2'], biases['bc2'])
conv2 = maxpool2d(conv2, k=2)
fc1 = tf.reshape(conv2, [-1, weights['wd1'].get_shape().as_list()[0]])
fc1 = tf.add(tf.matmul(fc1, weights['wd1']), biases['bd1'])
fc1 = tf.nn.relu(fc1)
fc1 = tf.nn.dropout(fc1, dropout)
out = tf.add(tf.matmul(fc1, weights['out']), biases['out'])
return out
# Initialize weights and biases
weights = {
'wc1': tf.Variable(tf.random_normal([5, 5, 1, 32])),
'wc2': tf.Variable(tf.random_normal([5, 5, 32, 64])),
'wd1': tf.Variable(tf.random_normal([7*7*64, 1024])),
'out': tf.Variable(tf.random_normal([1024, n_classes]))
}
biases = {
'bc1': tf.Variable(tf.random_normal([32])),
'bc2': tf.Variable(tf.random_normal([64])),
'bd1': tf.Variable(tf.random_normal([1024])),
'out': tf.Variable(tf.random_normal([n_classes]))
}
# Construct model
pred = conv_net(x, weights, biases, keep_prob)
# Define loss and optimizer
cost = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=pred, labels=y))
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cost)
# Evaluate model
correct_pred = tf.equal(tf.argmax(pred, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
```
接下来,我们可以开始训练模型,同时在训练过程中使用Tkinter创建一个可视化界面,用于展示模型的训练过程和识别结果。以下是完整的代码:
```
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
import tkinter as tk
from PIL import Image, ImageDraw
# Define parameters
learning_rate = 0.001
training_iters = 20000
batch_size = 128
display_step = 10
# Network parameters
n_input = 784
n_classes = 10
dropout = 0.75
# Create placeholders
x = tf.placeholder(tf.float32, [None, n_input])
y = tf.placeholder(tf.float32, [None, n_classes])
keep_prob = tf.placeholder(tf.float32)
# Create convnet
def conv2d(x, W, b, strides=1):
x = tf.nn.conv2d(x, W, strides=[1, strides, strides, 1], padding='SAME')
x = tf.nn.bias_add(x, b)
return tf.nn.relu(x)
def maxpool2d(x, k=2):
return tf.nn.max_pool(x, ksize=[1, k, k, 1], strides=[1, k, k, 1], padding='SAME')
def conv_net(x, weights, biases, dropout):
x = tf.reshape(x, shape=[-1, 28, 28, 1])
conv1 = conv2d(x, weights['wc1'], biases['bc1'])
conv1 = maxpool2d(conv1, k=2)
conv2 = conv2d(conv1, weights['wc2'], biases['bc2'])
conv2 = maxpool2d(conv2, k=2)
fc1 = tf.reshape(conv2, [-1, weights['wd1'].get_shape().as_list()[0]])
fc1 = tf.add(tf.matmul(fc1, weights['wd1']), biases['bd1'])
fc1 = tf.nn.relu(fc1)
fc1 = tf.nn.dropout(fc1, dropout)
out = tf.add(tf.matmul(fc1, weights['out']), biases['out'])
return out
# Initialize weights and biases
weights = {
'wc1': tf.Variable(tf.random_normal([5, 5, 1, 32])),
'wc2': tf.Variable(tf.random_normal([5, 5, 32, 64])),
'wd1': tf.Variable(tf.random_normal([7*7*64, 1024])),
'out': tf.Variable(tf.random_normal([1024, n_classes]))
}
biases = {
'bc1': tf.Variable(tf.random_normal([32])),
'bc2': tf.Variable(tf.random_normal([64])),
'bd1': tf.Variable(tf.random_normal([1024])),
'out': tf.Variable(tf.random_normal([n_classes]))
}
# Construct model
pred = conv_net(x, weights, biases, keep_prob)
# Define loss and optimizer
cost = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=pred, labels=y))
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cost)
# Evaluate model
correct_pred = tf.equal(tf.argmax(pred, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
# Start training
init = tf.global_variables_initializer()
sess = tf.Session()
sess.run(init)
step = 1
while step * batch_size < training_iters:
batch_x, batch_y = mnist.train.next_batch(batch_size)
sess.run(optimizer, feed_dict={x: batch_x, y: batch_y, keep_prob: dropout})
if step % display_step == 0:
acc = sess.run(accuracy, feed_dict={x: batch_x, y: batch_y, keep_prob: 1.})
print("Step " + str(step*batch_size) + ", Training Accuracy= " + "{:.5f}".format(acc))
step += 1
print("Optimization Finished!")
# Create Tkinter GUI
root = tk.Tk()
root.title("Mnist Digit Recognition")
# Create canvas for drawing
canvas_width = 200
canvas_height = 200
canvas = tk.Canvas(root, width=canvas_width, height=canvas_height, bg="white")
canvas.pack()
# Create PIL image for drawing
image = Image.new("L", (canvas_width, canvas_height), 0)
draw = ImageDraw.Draw(image)
# Define function for classifying drawn digit
def classify_digit():
# Resize image to 28x28
digit_image = image.resize((28, 28))
# Convert image to numpy array
digit_array = tf.keras.preprocessing.image.img_to_array(digit_image)
digit_array = digit_array.reshape((1, 784))
digit_array = digit_array.astype('float32')
digit_array /= 255
# Classify digit using trained model
prediction = sess.run(tf.argmax(pred, 1), feed_dict={x: digit_array, keep_prob: 1.})
# Display prediction
prediction_label.config(text="Prediction: " + str(prediction[0]))
# Define function for clearing canvas
def clear_canvas():
canvas.delete("all")
draw.rectangle((0, 0, canvas_width, canvas_height), fill=0)
# Create buttons and labels
classify_button = tk.Button(root, text="Classify", command=classify_digit)
classify_button.pack(side="top")
clear_button = tk.Button(root, text="Clear", command=clear_canvas)
clear_button.pack(side="top")
prediction_label = tk.Label(root, text="")
prediction_label.pack(side="bottom")
# Define canvas event handlers
def on_left_button_down(event):
canvas.bind("<B1-Motion>", on_mouse_move)
def on_left_button_up(event):
canvas.unbind("<B1-Motion>")
def on_mouse_move(event):
x, y = event.x, event.y
canvas.create_oval(x-10, y-10, x+10, y+10, fill="black")
draw.ellipse((x-10, y-10, x+10, y+10), fill=255)
canvas.bind("<Button-1>", on_left_button_down)
canvas.bind("<ButtonRelease-1>", on_left_button_up)
root.mainloop()
```
在训练过程中,程序会打印出每个batch的训练准确率。在训练完成后,程序会创建一个Tkinter窗口,包含一个用于绘制手写数字的画布、一个用于清除画布的按钮、一个用于识别手写数字并显示结果的按钮,以及一个用于显示识别结果的标签。
相关推荐
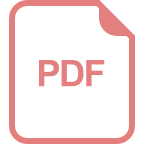








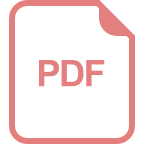