fragment如何隐藏在activity中设置的控件
时间: 2024-05-10 19:17:33 浏览: 10
在 Android 中,可以使用 Fragment 来隐藏 Activity 中设置的控件。具体步骤如下:
1. 在 Activity 的布局文件中,使用 `<fragment>` 标签来添加 Fragment。
2. 在 Fragment 的布局文件中,添加需要隐藏的控件。
3. 在 Fragment 中使用 `getView()` 方法获取 Fragment 的根视图,并使用 `setVisibility()` 方法将需要隐藏的控件设置为 `View.GONE`。
举个例子:
Activity 布局文件:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<fragment
android:id="@+id/my_fragment"
android:name="com.example.MyFragment"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<TextView
android:id="@+id/my_textview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:layout_centerInParent="true" />
</RelativeLayout>
```
Fragment 布局文件:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/hide_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hide TextView" />
</LinearLayout>
```
Fragment 代码:
```
public class MyFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_my, container, false);
Button hideButton = (Button) view.findViewById(R.id.hide_button);
hideButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
View textView = getView().findViewById(R.id.my_textview);
textView.setVisibility(View.GONE);
}
});
return view;
}
}
```
在上面的例子中,当用户点击 Fragment 中的按钮时,`TextView` 将被隐藏。
相关推荐
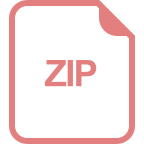
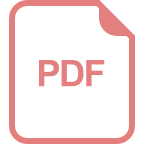
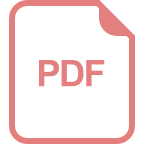














