具体python程序和stm32的程序
时间: 2024-03-14 12:46:38 浏览: 202
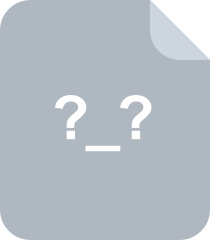
stm32程序例程
这里提供一个简单的Python程序和STM32程序的示例,实现Python向STM32发送一个字符串,STM32接收到字符串并将其返回给Python。
Python程序:
```python
import serial
ser = serial.Serial('COM3', 9600) # 初始化串口,COM3为串口号,9600为波特率
ser.write(b'Hello STM32') # 发送字符串
data = ser.read(10) # 接收返回的数据,10为接收数据的字节数
print(data) # 输出接收到的数据
```
STM32程序:
```c
#include "stm32f4xx.h"
#include "stdio.h"
#include "string.h"
#define RX_BUF_SIZE 10 // 接收缓冲区大小
char rx_buf[RX_BUF_SIZE]; // 接收缓冲区
int rx_idx = 0; // 接收缓冲区索引
void USART2_IRQHandler(void)
{
if (USART_GetITStatus(USART2, USART_IT_RXNE) != RESET) // 判断是否接收到数据
{
char c = USART_ReceiveData(USART2); // 读取接收到的数据
if (c == '\n' || rx_idx == RX_BUF_SIZE-1) // 判断是否接收到完整的字符串
{
rx_buf[rx_idx] = '\0'; // 在字符串结尾添加'\0',表示字符串结束
USART_SendData(USART2, (uint8_t*)rx_buf, strlen(rx_buf)); // 将接收到的字符串返回给Python
rx_idx = 0; // 重置接收缓冲区索引
}
else
{
rx_buf[rx_idx++] = c; // 将接收到的字符存入接收缓冲区
}
}
}
int main(void)
{
USART_InitTypeDef USART_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
USART_Cmd(USART2, ENABLE);
while(1)
{
}
}
```
在这个示例中,Python程序通过串口向STM32发送字符串"Hello STM32",STM32接收到字符串后,将其返回给Python。STM32程序中使用了中断方式接收串口数据,当接收到完整的字符串时,将其返回给Python。如果需要进行更复杂的数据交互,可以根据需要进行相应的修改。
阅读全文
相关推荐
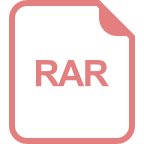
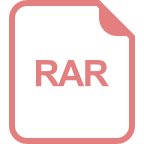
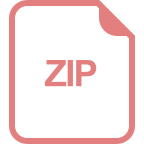
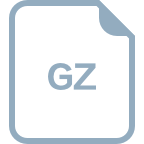
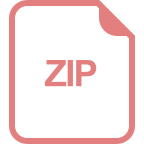










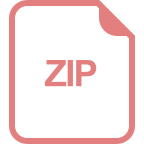
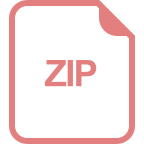
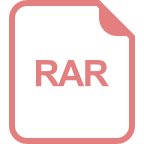