C语言,求一个大于10的n位整数的后n-1位的数,并作为函数值返回。使用int fun(int w)
时间: 2023-12-24 18:14:42 浏览: 385
好的,针对您的问题,可以使用以下代码实现:
```
int fun(int w) {
int n = 0, num = w;
while (num > 0) {
num /= 10;
n++;
}
if (n <= 1) {
return 0;
}
return w % (int)pow(10, n - 1);
}
```
该函数先计算出参数 `w` 的位数 `n`,如果 `n` 不大于 1,则返回 0。
否则,使用取余运算 `w % (int)pow(10, n - 1)` 得到 `w` 的后 `n - 1` 位数,并将其作为函数值返回。
需要注意的是,由于 `pow` 函数返回的是 `double` 类型,需要进行强制类型转换为 `int` 类型。
相关问题
用c语言编写函数fun,w是一个大于10的无符号函数,若w是n位的整数,则函数求出w的后n-1位的数作为函数值返回
在C语言中,我们可以创建一个名为`get_last_n_bits`的函数,它接受一个无符号整数`w`和一个整数`n`作为参数,然后通过位运算技巧获取并返回`w`的后`n-1`位。以下是相应的实现:
```c
#include <stdio.h>
#include <limits.h>
// 定义函数
unsigned long get_last_n_bits(unsigned w, int n) {
// 确保n大于等于2,因为最少需要一位来表示最低位
if (n < 2) {
printf("Error: n should be at least 2.\n");
return ULONG_MAX; // 返回一个特殊的值,表示错误
}
// 使用位左移操作,移动w的高位(n-1位)到最低位
unsigned mask = (1ull << n) - 1;
return w & mask;
}
int main() {
unsigned w = 27389; // 示例无符号整数
int n = 3; // 获取后三位
unsigned last_n_bits = get_last_n_bits(w, n);
printf("The last %d bits of %u are: %lu\n", n - 1, w, last_n_bits);
return 0;
}
```
上述代码中,`get_last_n_bits`函数首先检查`n`是否足够大,如果不够则返回一个特殊值表示错误。接着,它使用按位与(bitwise AND)操作符`&`,结合掩码`mask`,得到`w`的最后`n-1`位。
用C语言编写一个函数unsigned fun (unsigned w),w是一个大于10的无符号整数,若w是n(n≥2)位的整数,函数求出w的地n-1位的数作为函数值返回。
旋转编码器是一种用于测量旋转运动的传感器,它可以输出旋转方向和旋转量。在51单片机中,可以通过外部中断和定时器来驱动旋转编码器。
以下是一个简单的51单片机驱动旋转编码器的程序:
```
#include<reg51.h>
sbit EN=P3^0; //使能脚
sbit DT=P3^1; //方向脚
sbit CLK=P3^2; //时钟脚
unsigned char code KeyCharTable[]={"0123456789abcdef"}; //显示字符表
unsigned char xdata SpeedBuffer[4]; //速度缓存
unsigned int xdata Speed; //速度值
unsigned char xdata Count; //计数器
unsigned char xdata Direction; //方向标志
void main()
{
EA=1; //开总中断
EN=1; //使能脚置高
while(1)
{
//显示速度值
Speed=SpeedBuffer[0]*1000+SpeedBuffer[1]*100+SpeedBuffer[2]*10+SpeedBuffer[3];
P0=KeyCharTable[Speed/1000];
P2=0x7f;
P2=0xff;
P0=KeyCharTable[Speed%1000/100];
P2=0xbf;
P2=0xff;
P0=KeyCharTable[Speed%100/10];
P2=0xdf;
P2=0xff;
P0=KeyCharTable[Speed%10];
P2=0xef;
P2=0xff;
}
}
void Timer0() interrupt 1
{
unsigned char xdata i;
Count++; //计数器加1
if(Count==50) //计数器到50时更新速度值
{
Speed=0;
for(i=0;i<4;i++)
{
Speed+=SpeedBuffer[i]*10^i;
}
Count=0;
for(i=3;i>0;i--) //速度缓存右移一位
{
SpeedBuffer[i]=SpeedBuffer[i-1];
}
SpeedBuffer[0]=0; //清零最高位
}
}
void ExternalInterrupt0() interrupt 0
{
if(DT==CLK) //判断方向
{
Direction=1; //正向
}
else
{
Direction=0; //反向
}
if(Direction==1) //正向计数
{
SpeedBuffer[0]++;
if(SpeedBuffer[0]==10) //最低位计满
{
SpeedBuffer[0]=0; //清零最低位
SpeedBuffer[1]++; //进位
if(SpeedBuffer[1]==10) //第二位计满
{
SpeedBuffer[1]=0; //清零第二位
SpeedBuffer[2]++; //进位
if(SpeedBuffer[2]==10) //第三位计满
{
SpeedBuffer[2]=0; //清零第三位
SpeedBuffer[3]++; //进位
}
}
}
}
else //反向计数
{
SpeedBuffer[0]--;
if(SpeedBuffer[0]==255) //最低位借位
{
SpeedBuffer[0]=9; //借位
SpeedBuffer[1]--; //退位
if(SpeedBuffer[1]==255) //第二位借位
{
SpeedBuffer[1]=9; //借位
SpeedBuffer[2]--; //退位
if(SpeedBuffer[2]==255) //第三位借位
{
SpeedBuffer[2]=9; //借位
SpeedBuffer[3]--; //退位
}
}
}
}
}
```
在这个程序中,使用了一个计数器来统计旋转编码器的旋转量,每50次中断更新一次速度值。在外部中断中判断旋转方向,并根据方向进行计数。最后将速度值转换为字符并显示在数码管上。
需要注意的是,旋转编码器的引脚需要连接到51单片机的外部中断0和定时器0的时钟输入引脚,同时需要在代码中对引脚进行定义和配置。
阅读全文
相关推荐
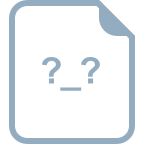
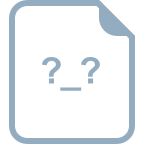













