使用JAVA代码编写一个日记本功能的程序,使用字节流经日记的具体信息记录在本地的txt文件中。当用户输入日记的特定内容后,会将输入的内容保存至本地的txt文件中。需要输入的内容包括“姓名”,“天气”、“标题”、“内容”的数据。保存的时候需要判断本地是否存在文件,如果存在则追加,不存在则新建。文件命名格式为“黑马日记本”加上“.txt”后缀,如“黑马日记本.txt”
时间: 2023-09-16 10:08:49 浏览: 211
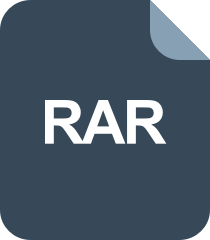
一个用JAVA编写的记事本.rar_java 记事本_java小记事本_用Java做一个记_记事本 java_记事本程序

以下是一个示例代码,实现了上述功能:
```java
import java.io.*;
public class Diary {
public static void main(String[] args) {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("请输入姓名:");
String name = null;
try {
name = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.print("请输入天气:");
String weather = null;
try {
weather = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.print("请输入标题:");
String title = null;
try {
title = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.print("请输入内容:");
String content = null;
try {
content = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
String fileName = "黑马日记本.txt";
File file = new File(fileName);
try {
FileWriter writer = new FileWriter(file, true);
writer.write("姓名:" + name + "\n");
writer.write("天气:" + weather + "\n");
writer.write("标题:" + title + "\n");
writer.write("内容:" + content + "\n");
writer.write("--------------------\n");
writer.close();
System.out.println("保存成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该程序先通过 `BufferedReader` 从控制台读取用户输入的姓名、天气、标题和内容,然后将这些信息写入指定的文件中。文件名为“黑马日记本.txt”,如果文件不存在则会自动创建。同时,使用 `FileWriter` 的构造方法中的 `true` 参数表示以追加的方式写入文件,即如果文件已存在,则在文件末尾追加内容,否则创建新文件。文件中每条日记之间用 `"--------------------\n"` 分隔。
注意,这只是一个简单的示例程序,实际应用中还需要进行错误处理等其他操作。
阅读全文
相关推荐
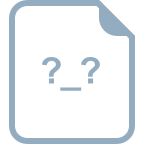
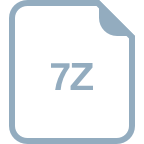
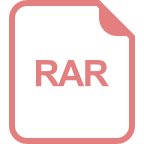
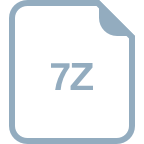
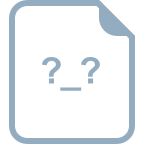
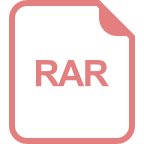
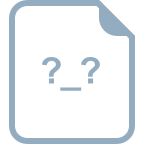
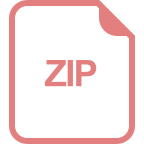
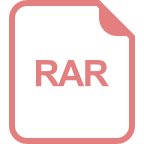
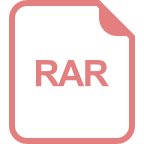
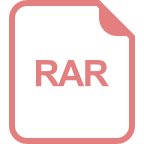
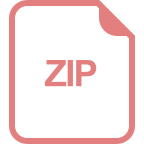
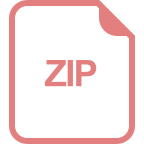
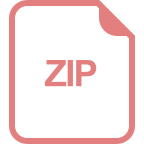
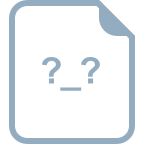
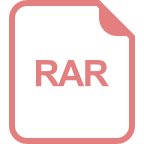