Android studio如何设计计算器
时间: 2024-05-26 09:10:36 浏览: 14
Android Studio可以使用Java语言来设计计算器。
首先,创建一个XML布局文件来定义计算器UI的外观。可以使用按钮、文本框和一些其他UI元素。
接下来,通过Java代码实现计算器的逻辑,例如使用事件监听器来处理按钮的点击事件,并通过计算表达式来计算结果,最后将结果显示在文本框中。
如果需要更加复杂的计算器功能,可以使用更高级的数学库或者添加更多的操作按钮。
相关问题
android studio设计计算器
好的,下面是一个简单的Android Studio设计计算器的步骤:
1. 打开Android Studio并创建一个新的空项目。
2. 在设计视图中,添加一个EditText和一个GridLayout作为布局。
3. 将GridLayout中的按钮逐一添加到布局中。您可以使用按钮作为操作符,数字和其他功能(例如清除和等于)。
4. 在EditText中,您将显示计算器的输出。您可以使用setText()方法将值设置为EditText中的文本。
5. 为每个按钮设置onClick()方法。这些方法将执行特定操作,并在EditText中显示结果。
6. 在设计完成后,请运行应用程序并测试您的计算器是否按预期工作。
希望这些步骤可以帮助您设计一个简单的计算器。
android studio设计计算器复数或非运算
以下是使用Android Studio设计计算器的示例代码,用于进行复数或非运算:
复数运算:
```java
public class Complex {
private double real, imaginary;
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public double getReal() {
return real;
}
public double getImaginary() {
return imaginary;
}
public Complex add(Complex other) {
double resultReal = this.real + other.getReal();
double resultImaginary = this.imaginary + other.getImaginary();
return new Complex(resultReal, resultImaginary);
}
public Complex subtract(Complex other) {
double resultReal = this.real - other.getReal();
double resultImaginary = this.imaginary - other.getImaginary();
return new Complex(resultReal, resultImaginary);
}
public Complex multiply(Complex other) {
double resultReal = this.real * other.getReal() - this.imaginary * other.getImaginary();
double resultImaginary = this.real * other.getImaginary() + this.imaginary * other.getReal();
return new Complex(resultReal, resultImaginary);
}
public Complex divide(Complex other) {
double denominator = other.getReal() * other.getReal() + other.getImaginary() * other.getImaginary();
double resultReal = (this.real * other.getReal() + this.imaginary * other.getImaginary()) / denominator;
double resultImaginary = (this.imaginary * other.getReal() - this.real * other.getImaginary()) / denominator;
return new Complex(resultReal, resultImaginary);
}
public String toString() {
String result = "";
if (real != 0) {
result += real;
}
if (imaginary > 0) {
result += "+" + imaginary + "i";
} else if (imaginary < 0) {
result += imaginary + "i";
}
if (result.equals("")) {
result = "0";
}
return result;
}
}
```
非运算:
```java
public class Not {
public static boolean not(boolean value) {
return !value;
}
}
```
这些代码可以在Android Studio中的Java文件中使用,例如一个名为"MainActivity.java"的文件。在此文件中,您可以创建UI元素,例如按钮和文本视图,然后将它们与上述代码连接,以便当用户点击按钮时执行所需的计算操作。
相关推荐
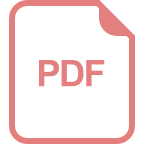
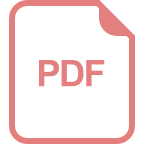












