使用mabatis-plus实现, 列表的查询需实现分页查询,可模糊查询图书名称、版号、出版社, 可按价格区间查询图书定价
时间: 2024-02-06 17:02:04 浏览: 127
好的,这是一个Java后端开发的问题。使用Mybatis-Plus实现分页查询和模糊查询是非常常见的需求。以下是一个示例:
```java
// 导入相关的类
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookService {
@Autowired
private BookMapper bookMapper;
public List<Book> searchBooks(String name, String edition, String publisher, Double minPrice, Double maxPrice, Integer pageNum, Integer pageSize) {
// 创建一个分页对象
Page<Book> page = new Page<>(pageNum, pageSize);
// 创建一个查询条件的包装器
QueryWrapper<Book> wrapper = new QueryWrapper<>();
// 实现模糊查询
if (name != null && !name.isEmpty()) {
wrapper.like("name", name);
}
if (edition != null && !edition.isEmpty()) {
wrapper.like("edition", edition);
}
if (publisher != null && !publisher.isEmpty()) {
wrapper.like("publisher", publisher);
}
// 实现价格区间查询
if (minPrice != null && maxPrice != null) {
wrapper.between("price", minPrice, maxPrice);
}
// 调用Mybatis-Plus的分页查询方法
bookMapper.selectPage(page, wrapper);
// 返回查询结果
return page.getRecords();
}
}
```
上面的代码中,我们使用了Mybatis-Plus提供的分页对象Page和查询条件包装器QueryWrapper,来实现分页查询和模糊查询。其中,like方法实现模糊查询,between方法实现价格区间查询。在查询方法中,我们调用了Mybatis-Plus提供的selectPage方法来实现分页查询。最后,我们返回查询结果。
希望这个示例可以帮助您完成您的需求。
阅读全文
相关推荐
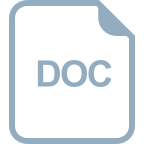
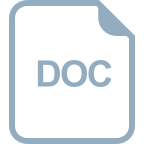
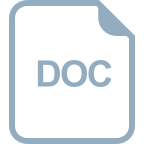

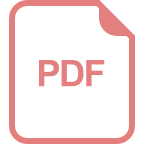
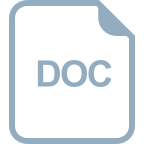





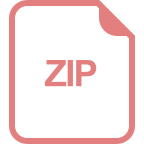
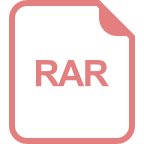
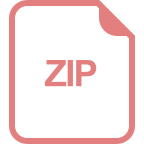




