uniapp的组件如何在pages.json文件中注册
时间: 2023-12-04 15:05:41 浏览: 37
在uniapp中,我们需要在pages.json文件中注册组件,才能在应用中使用它们。下面是一个简单的示例:
1. 在pages.json文件中找到"pages"节点,添加一个新的节点"usingComponents",用于注册组件。
```json
{
"pages": [
{
"path": "pages/index/index",
"style": {
"navigationBarTitleText": "首页"
}
}
],
"usingComponents": {
"custom-component": "/components/custom-component/custom-component"
}
}
```
2. 在"usingComponents"节点中,添加一个新的键值对,其中键是组件的名称,值是组件的路径。这里我们以"custom-component"组件为例,路径为"/components/custom-component/custom-component"。
3. 接下来,在页面的wxml文件中直接使用组件即可。
```html
<custom-component></custom-component>
```
这样,我们就可以在uniapp应用中使用自定义组件了。需要注意的是,组件的路径应该是相对于pages.json文件的路径。如果组件在项目的根目录下,则路径应该是以"/"开头的绝对路径。
相关问题
uniapp怎么在pages.json的tabBar里面做中英文切换
要在uniapp的pages.json的tabBar里面实现中英文切换,可以按照以下步骤进行操作:
1. 在pages.json里面添加一个lang字段,用来保存当前语言的信息,如下所示:
```json
{
"pages": [
{
"path": "pages/index/index",
"name": "index",
"lang": "zh", // 当前语言为中文
"text": "首页",
"iconPath": "static/tabbar/home.png",
"selectedIconPath": "static/tabbar/home-active.png"
},
// 其他tabBar页面
],
"tabBar": {
// tabBar配置信息
}
}
```
2. 在app.vue里面添加一个langChange方法,用来切换当前语言,并更新pages.json里面的lang字段,代码如下:
```vue
<template>
<view class="container">
<router-view />
</view>
</template>
<script>
export default {
data() {
return {
lang: "zh" // 初始化当前语言为中文
};
},
methods: {
langChange() {
this.lang = this.lang === "zh" ? "en" : "zh"; // 切换当前语言
uni.setStorageSync("lang", this.lang); // 将当前语言保存到本地缓存中
const pages = getCurrentPages(); // 获取当前页面栈
const len = pages.length;
for (let i = 0; i < len; i++) {
const page = pages[i];
if (page.route.indexOf("pages/") === 0) {
const route = page.route.replace("pages/", "");
const tabBar = this.$pagesJson.tabBar;
const index = tabBar.list.findIndex(item => item.pagePath === route);
if (index >= 0) {
tabBar.list[index].lang = this.lang; // 更新pages.json里面的lang字段
}
}
}
}
}
};
</script>
```
3. 在tabBar组件里面添加一个点击事件,调用langChange方法进行语言切换,代码如下:
```vue
<template>
<view class="tabbar">
<view
v-for="(item, index) in tabBar.list"
:key="index"
@click="switchTab(item.pagePath)"
:class="{ active: activeIndex === index }"
>
<image :src="activeIndex === index ? item.selectedIconPath : item.iconPath" />
<text>{{ item.text }}</text>
</view>
</view>
</template>
<script>
export default {
data() {
return {
activeIndex: 0 // 当前选中的tabBar项
};
},
methods: {
switchTab(pagePath) {
const tabBar = this.$pagesJson.tabBar;
const index = tabBar.list.findIndex(item => item.pagePath === pagePath);
if (index >= 0) {
this.activeIndex = index; // 更新当前选中的tabBar项
uni.switchTab({ url: `/${pagePath}` });
}
},
langChange() {
this.$parent.langChange(); // 调用父组件的langChange方法进行语言切换
}
}
};
</script>
```
4. 在需要进行中英文切换的页面里面,根据lang字段来显示对应的中英文文本,代码如下:
```vue
<template>
<view class="container">
<text>{{ lang === "zh" ? "中文文本" : "English Text" }}</text>
</view>
</template>
<script>
export default {
computed: {
lang() {
return this.$page.meta.lang; // 获取当前页面的lang字段
}
}
};
</script>
```
这样,就可以在uniapp的pages.json的tabBar里面实现中英文切换了。
uniapp动态生成page.json
uniapp是一种基于Vue.js框架的前端开发框架,它可以同时在各种平台上创建高性能的本地应用。在uniapp中,Page.json是一个非常重要的文件,它描述了页面的一些属性,例如页面的路径、页面的颜色和页面的文字样式等。在uniapp中,我们可以通过使用JavaScript动态生成Page.json文件,从而允许我们在完全运行时生成页面。
首先,我们需要在uniapp中定义一个全局的变量config,并将它注册到Vue.prototype上。在config中,我们可以定义一些默认的页面属性。例如:
```
var config = {
pages: [
{
path: 'pages/index/index',
style: {
navigationBarTitleText: '首页'
}
},
{
path: 'pages/about/about',
style: {
navigationBarTitleText: '关于我们'
}
}
]
}
Vue.prototype.$config = config;
```
然后,我们就可以在我们的Vue组件中使用这个全局变量了。我们可以通过在mounted中动态生成Page.json文件。
```
module.exports = {
mounted() {
var pages = this.$config.pages;
var jsonStr = JSON.stringify({ "pages": pages });
uni.writeFile({
filePath: 'pages.json',
data: jsonStr,
success() {
console.log('生成页面json成功')
},
fail(e) {
console.log('生成页面json失败', e)
}
});
},
}
```
在上面的代码中,我们首先获取了我们定义的config的pages属性。然后,我们将这个pages转换为JSON格式并将其写入到pages.json文件中。如果生成pages.json文件成功,则会打印“生成页面json成功”,否则会打印“生成页面json失败”。
通过上述方法,我们可以成功地动态生成Page.json文件,并且可以在完全运行时动态生成页面。当用户访问某个页面时,uniapp会根据Page.json文件创建并渲染对应的页面。我们可以通过动态生成的Page.json来改变页面的一些样式和属性,从而实现更加丰富和灵活的页面设计。
相关推荐
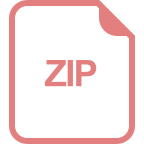
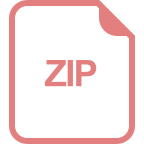
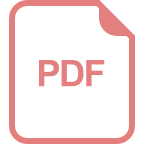












