springboot集成mybatisplus
时间: 2023-09-05 16:14:00 浏览: 112
好的,对于你的问题,我可以给出以下步骤:
1. 在pom.xml文件中添加如下的依赖:
```
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.3.1</version>
</dependency>
```
2. 在application.properties文件中配置数据源信息:
```
spring.datasource.url=jdbc:mysql://localhost:3306/db_name?useUnicode=true&characterEncoding=UTF-8&allowMultiQueries=true&useSSL=false&serverTimezone=GMT%2B8
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.username=username
spring.datasource.password=password
```
3. 创建MybatisPlusConfig.java类,包含以下代码:
```java
@Configuration
@MapperScan("com.example.demo.mapper")
public class MybatisPlusConfig {
// 配置逻辑删除插件
@Bean
public ISqlInjector sqlInjector() {
return new LogicSqlInjector();
}
// 分页插件
@Bean
public PaginationInterceptor paginationInterceptor() {
return new PaginationInterceptor();
}
}
```
4. 创建需要操作的实体类和Mapper接口,以User类和UserMapper接口为例:
User实体类示例:
```java
@Data
public class User implements Serializable {
private static final long serialVersionUID = 1L;
@TableId(value = "id", type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
private String email;
private LocalDateTime createTime;
private LocalDateTime updateTime;
@TableLogic
private Integer deleted;
}
```
UserMapper接口示例:
```java
public interface UserMapper extends BaseMapper<User> {
}
```
5. 在Controller中注入UserMapper并进行相关操作即可完成集成。示例代码:
```java
@RestController
public class UserController {
@Autowired
private UserMapper userMapper;
@GetMapping("/getUser")
public User getUser(@RequestParam("id") Long id) {
return userMapper.selectById(id);
}
@PostMapping("/insertUser")
public String insertUser(@RequestBody User user) {
int result = userMapper.insert(user);
return result > 0 ? "success" : "fail";
}
}
```
希望以上回答对你有帮助。如果你有其他问题,可以随时提出来哦!
阅读全文
相关推荐
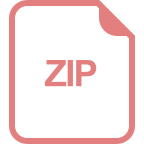

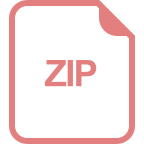
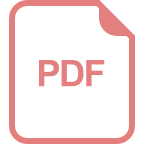
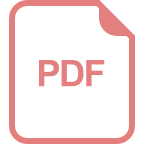
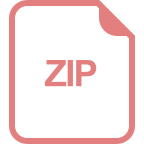
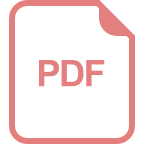
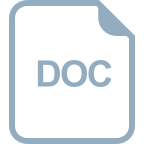
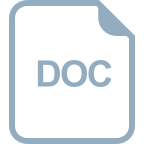




