用c语言编写一个高速缓存模拟程序
时间: 2023-09-06 21:13:56 浏览: 114
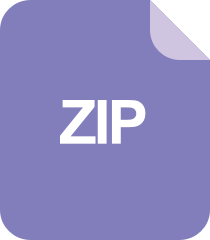
基于C语言的高速缓冲器模拟.zip

好的,下面是一个简单的高速缓存模拟程序的示例代码,其中使用了结构体来表示缓存块和缓存集:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define CACHE_SIZE 16 // 缓存大小
#define BLOCK_SIZE 64 // 块大小
#define NUM_SETS CACHE_SIZE / 4 // 集合数量
typedef struct {
bool valid; // 是否有效
int tag; // 标记位
char data[BLOCK_SIZE]; // 块数据
} cache_block;
typedef struct {
cache_block blocks[4]; // 每个集合包含4个块
} cache_set;
cache_set cache[NUM_SETS]; // 缓存
int main() {
FILE* fp = fopen("test.txt", "rb"); // 以二进制方式打开文件
if (!fp) {
printf("Failed to open file.\n");
return 1;
}
int hits = 0, misses = 0; // 命中次数和未命中次数
// 读取文件中的数据
while (!feof(fp)) {
int address;
fread(&address, sizeof(int), 1, fp); // 读取地址
int set_index = (address / BLOCK_SIZE) % NUM_SETS; // 计算集合索引
int tag = address / (BLOCK_SIZE * NUM_SETS); // 计算标记位
bool hit = false;
for (int i = 0; i < 4; i++) {
cache_block* block = &cache[set_index].blocks[i];
if (block->valid && block->tag == tag) { // 命中
hit = true;
hits++;
break;
}
}
if (!hit) { // 未命中
misses++;
int block_index = rand() % 4; // 随机替换一个块
cache_block* block = &cache[set_index].blocks[block_index];
block->valid = true;
block->tag = tag;
fseek(fp, address, SEEK_SET); // 定位到地址处
fread(&block->data, sizeof(char), BLOCK_SIZE, fp); // 读取块数据
}
}
printf("Hits: %d, Misses: %d\n", hits, misses);
fclose(fp);
return 0;
}
```
这个程序模拟了一个4路组相联的高速缓存,每个集合包含4个块,使用随机替换算法进行替换。在读取文件中的数据时,先计算出地址所属的集合索引和标记位,然后在缓存中查找该块是否已经被缓存。如果已经被缓存,则命中;否则未命中,需要从文件中读取块数据,并将其存入缓存中。最终输出命中次数和未命中次数。
阅读全文
相关推荐
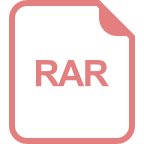
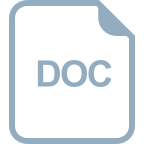

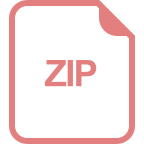
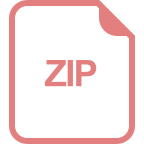
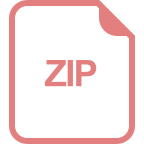
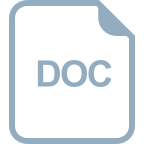
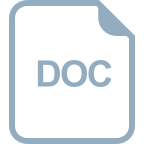
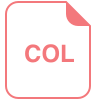
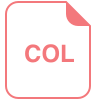




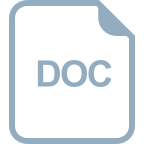
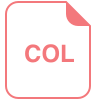