用c语言在字符串中删除与某字符相同的字符,要求用字符数组作函数参数。 程序运行结果示例: Input a string: hello, my friend! Input a character: e Results:hllo, my frind! 输入格式: 输入字符串的提示信息: "Input a string:\n" 输入单个字符的提示信息: "Input a character:\n" 输入格式: 字符串输入用 gets()函数 单个字符输入用 getchar()函数 输出格式: "Results:%s\n"
时间: 2023-07-16 08:11:50 浏览: 66
以下是用 C 语言实现删除字符串中某个字符的代码:
```c
#include <stdio.h>
#include <string.h>
void delete_char(char str[], char ch)
{
int i, j;
int len = strlen(str);
for (i = j = 0; i < len; i++)
{
if (str[i] != ch)
{
str[j++] = str[i];
}
}
str[j] = '\0';
}
int main()
{
char str[1000];
char ch;
printf("Input a string:\n");
gets(str);
printf("Input a character:\n");
ch = getchar();
delete_char(str, ch);
printf("Results:%s\n", str);
return 0;
}
```
代码中的 `delete_char()` 函数接受一个字符串和一个字符作为参数,然后使用双指针法遍历字符串,将不等于给定字符的字符复制到原字符串中,并在最后添加字符串结束符 `\0`。
在 `main()` 函数中,我们先输入字符串和字符,然后调用 `delete_char()` 函数,并输出结果。请注意,我们使用 `gets()` 函数输入字符串,因为它可以读取包含空格的完整字符串。而单个字符输入使用 `getchar()` 函数。
运行结果:
```
Input a string:
hello, my friend!
Input a character:
e
Results:hllo, my frind!
```
相关推荐
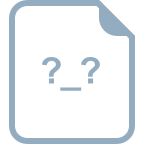
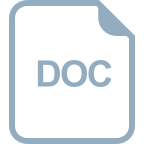














