用android和bmob实现外卖购物车(listview中的数据从bomb中获取的,有金额总和,左右有加减按钮,点击加号时数量加一)详细代码
时间: 2024-01-24 16:18:38 浏览: 30
这里是一个简单的使用 Android 和 Bmob 实现外卖购物车的代码示例。以下是实现步骤:
1. 创建一个名为 `OrderItem` 的 Bmob 数据库表格,用于存储外卖订单的详细信息,包括商品名称、价格和数量等。
2. 在 Android Studio 中创建一个名为 `OrderAdapter` 的 Adapter 类,用于显示订单列表中的每个订单项。
```java
public class OrderAdapter extends ArrayAdapter<OrderItem> {
private ArrayList<OrderItem> orderItems;
public OrderAdapter(Context context, int textViewResourceId, ArrayList<OrderItem> orderItems) {
super(context, textViewResourceId, orderItems);
this.orderItems = orderItems;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View v = convertView;
if (v == null) {
LayoutInflater vi = (LayoutInflater) getContext().getSystemService(Context.LAYOUT_INFLATER_SERVICE);
v = vi.inflate(R.layout.order_item, null);
}
OrderItem orderItem = orderItems.get(position);
if (orderItem != null) {
TextView nameTextView = (TextView) v.findViewById(R.id.nameTextView);
TextView priceTextView = (TextView) v.findViewById(R.id.priceTextView);
TextView quantityTextView = (TextView) v.findViewById(R.id.quantityTextView);
Button addButton = (Button) v.findViewById(R.id.addButton);
Button subtractButton = (Button) v.findViewById(R.id.subtractButton);
if (nameTextView != null) {
nameTextView.setText(orderItem.getName());
}
if (priceTextView != null) {
priceTextView.setText("$" + orderItem.getPrice());
}
if (quantityTextView != null) {
quantityTextView.setText(Integer.toString(orderItem.getQuantity()));
}
if (addButton != null) {
addButton.setTag(position);
addButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int position = (Integer) view.getTag();
OrderItem orderItem = orderItems.get(position);
orderItem.setQuantity(orderItem.getQuantity() + 1);
notifyDataSetChanged();
}
});
}
if (subtractButton != null) {
subtractButton.setTag(position);
subtractButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int position = (Integer) view.getTag();
OrderItem orderItem = orderItems.get(position);
if (orderItem.getQuantity() > 0) {
orderItem.setQuantity(orderItem.getQuantity() - 1);
notifyDataSetChanged();
}
}
});
}
}
return v;
}
}
```
3. 在 `MainActivity` 中,从 Bmob 数据库中获取订单列表并显示在 `ListView` 中。同时,计算购物车中所有订单的总金额。
```java
public class MainActivity extends AppCompatActivity {
private ListView orderListView;
private TextView totalTextView;
private ArrayList<OrderItem> orderItems;
private OrderAdapter orderAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
orderListView = (ListView) findViewById(R.id.orderListView);
totalTextView = (TextView) findViewById(R.id.totalTextView);
orderItems = new ArrayList<OrderItem>();
orderAdapter = new OrderAdapter(this, R.layout.order_item, orderItems);
orderListView.setAdapter(orderAdapter);
BmobQuery<OrderItem> query = new BmobQuery<OrderItem>();
query.findObjects(new FindListener<OrderItem>() {
@Override
public void done(List<OrderItem> list, BmobException e) {
if (e == null) {
orderItems.addAll(list);
orderAdapter.notifyDataSetChanged();
double total = 0;
for (OrderItem orderItem : orderItems) {
total += orderItem.getPrice() * orderItem.getQuantity();
}
totalTextView.setText("$" + total);
}
}
});
}
}
```
4. 最后,创建 `order_item.xml` 布局文件,用于显示每个订单项的名称、价格、数量和加减按钮。
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/nameTextView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Name" />
<TextView
android:id="@+id/priceTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="$0" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/subtractButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="-" />
<TextView
android:id="@+id/quantityTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0" />
<Button
android:id="@+id/addButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="+" />
</LinearLayout>
</LinearLayout>
```
这样就完成了使用 Android 和 Bmob 实现外卖购物车的代码示例。
相关推荐
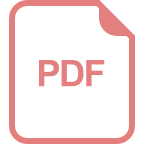
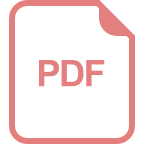














