stringRedisTemplate获取redis中hash的全部值,每次批量获取1000个,怎么写
时间: 2024-03-20 21:45:19 浏览: 17
你可以使用Redis的`scan`命令来实现分批获取hash中的全部值,具体步骤如下:
1. 使用`scan`命令获取hash中的全部field和value,每次获取1000个。可以使用`HashOperations`的`scan`方法来实现。
```
ScanOptions options = ScanOptions.scanOptions().count(1000).build();
Cursor<Map.Entry<Object, Object>> cursor = hashOperations.scan("hashKey", options);
```
2. 遍历游标,将field和value保存到一个Map中。
```
Map<Object, Object> resultMap = new HashMap<>();
while (cursor.hasNext()) {
Map.Entry<Object, Object> entry = cursor.next();
resultMap.put(entry.getKey(), entry.getValue());
}
```
3. 判断游标是否已经结束,如果未结束,则继续获取下一个批次的数据。如果已经结束,则返回保存了全部值的Map。
```
if (cursor.isClosed()) {
return resultMap;
} else {
options = options.cursor(cursor);
cursor = hashOperations.scan("hashKey", options);
}
```
完整代码示例如下:
```
public Map<Object, Object> getAllHashValues(String hashKey) {
HashOperations<String, Object, Object> hashOperations = stringRedisTemplate.opsForHash();
ScanOptions options = ScanOptions.scanOptions().count(1000).build();
Cursor<Map.Entry<Object, Object>> cursor = hashOperations.scan(hashKey, options);
Map<Object, Object> resultMap = new HashMap<>();
while (cursor.hasNext()) {
Map.Entry<Object, Object> entry = cursor.next();
resultMap.put(entry.getKey(), entry.getValue());
}
if (cursor.isClosed()) {
return resultMap;
} else {
options = options.cursor(cursor);
cursor = hashOperations.scan(hashKey, options);
while (cursor.hasNext()) {
Map.Entry<Object, Object> entry = cursor.next();
resultMap.put(entry.getKey(), entry.getValue());
}
return resultMap;
}
}
```
请注意,以上代码仅供参考,可能需要根据你的具体需求进行修改。
相关推荐
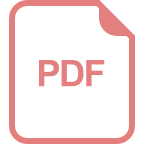
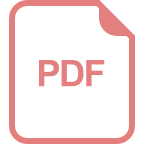
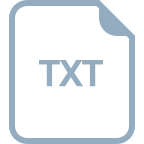














