c语言输入三个数从小到大排序输出要求用函数和指针实现
时间: 2023-12-10 22:41:04 浏览: 105
下面是用函数和指针实现输入三个数从小到大排序输出的C语言代码:
```c
#include <stdio.h>
void sort(int *a, int *b, int *c) {
int temp;
if (*a > *b) {
temp = *a;
*a = *b;
*b = temp;
}
if (*a > *c) {
temp = *a;
*a = *c;
*c = temp;
}
if (*b > *c) {
temp = *b;
*b = *c;
*c = temp;
}
}
int main() {
int a, b, c;
printf("请输入三个数:");
scanf("%d%d%d", &a, &b, &c);
sort(&a, &b, &c);
printf("从小到大排序后的结果为:%d %d %d\n", a, b, c);
return 0;
}
```
首先在主函数中使用 `scanf` 函数输入三个数,然后调用 `sort` 函数进行排序。 `sort` 函数传入三个指针,利用指针访问和修改变量的值,实现对三个数的排序。最后在主函数中输出排序后的结果。
相关问题
c语言输入三个字符串从小到大排序要求用函数和指针输出
下面是一个用 C 语言编写的程序,可以输入三个字符串并按字典序从小到大排序。
```c
#include <stdio.h>
#include <string.h>
void sort(char **p1, char **p2, char **p3);
int main()
{
char str1[100], str2[100], str3[100];
char *p1, *p2, *p3;
printf("请输入三个字符串:\n");
scanf("%s%s%s", str1, str2, str3);
p1 = str1;
p2 = str2;
p3 = str3;
sort(&p1, &p2, &p3);
printf("排序后的结果为:\n");
printf("%s\n%s\n%s\n", p1, p2, p3);
return 0;
}
void sort(char **p1, char **p2, char **p3)
{
char *tmp;
if (strcmp(*p1, *p2) > 0)
{
tmp = *p1;
*p1 = *p2;
*p2 = tmp;
}
if (strcmp(*p1, *p3) > 0)
{
tmp = *p1;
*p1 = *p3;
*p3 = tmp;
}
if (strcmp(*p2, *p3) > 0)
{
tmp = *p2;
*p2 = *p3;
*p3 = tmp;
}
}
```
在这个程序中,sort 函数接收三个指向字符数组的指针,然后使用 strcmp 函数比较字符串的大小,从而将它们按照字典序从小到大排序。在主函数中,我们先定义三个字符数组和三个指向字符数组的指针,并使用 scanf 函数读入三个字符串。然后将这三个指针传递给 sort 函数进行排序,并最终输出排序后的结果。
用指针变量的方法c语言输入三个整数从小到大排序
好的,以下是一个使用指针变量的方法,实现输入三个整数从小到大排序的C语言代码示例:
```c
#include <stdio.h>
void sort(int *a, int *b, int *c) {
int temp;
if (*a > *b) {
temp = *a;
*a = *b;
*b = temp;
}
if (*a > *c) {
temp = *a;
*a = *c;
*c = temp;
}
if (*b > *c) {
temp = *b;
*b = *c;
*c = temp;
}
}
int main() {
int a, b, c;
printf("请输入三个整数:");
scanf("%d%d%d", &a, &b, &c);
sort(&a, &b, &c);
printf("从小到大排序:%d %d %d\n", a, b, c);
return 0;
}
```
在这个代码中,我们首先定义了三个整数变量:a、b、c。然后,通过`scanf`语句,从用户输入中获取这三个整数的值。
接下来,我们定义了一个名为`sort`的函数,用于对三个数进行排序。这个函数有三个参数,分别是指向a、b、c三个变量的指针。在函数中,我们使用指针变量和临时变量temp来交换变量的值,实现了对三个数的排序。
最后,在主函数中,我们调用了`sort`函数,并将a、b、c三个变量的地址传递给了函数。最后,我们使用`printf`语句将排序后的结果输出到屏幕上。
需要注意的是,在调用`sort`函数时,我们需要传递a、b、c三个变量的地址,即使用`&`符号来取地址。
阅读全文
相关推荐
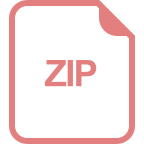













