stm32f103c6t6小游戏程序
时间: 2023-07-24 12:25:46 浏览: 40
好的,以下是一个简单的STM32F103C6T6小游戏程序示例,主要功能是控制LED灯的闪烁速度。这个游戏的规则是,当你按下按钮时,LED灯会开始闪烁,然后你需要尽可能快地再次按下按钮,以在LED灯闪烁时停止它。
首先,应该导入所需的库文件和头文件:
```c
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
```
然后,定义一些常量和变量:
```c
#define BUTTON_PIN GPIO_Pin_0
#define BUTTON_GPIO GPIOA
#define BUTTON_GPIO_CLK RCC_APB2Periph_GPIOA
#define LED_PIN GPIO_Pin_8
#define LED_GPIO GPIOB
#define LED_GPIO_CLK RCC_APB2Periph_GPIOB
int button_pressed = 0;
int led_on = 0;
int button_debounce = 0;
```
这里,我们定义了按钮和LED灯所连接的引脚,以及一些变量来跟踪游戏的状态。
接下来,我们需要初始化GPIO和定时器:
```c
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable GPIOA clock */
RCC_APB2PeriphClockCmd(BUTTON_GPIO_CLK, ENABLE);
/* Configure Button pin as input floating */
GPIO_InitStructure.GPIO_Pin = BUTTON_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(BUTTON_GPIO, &GPIO_InitStructure);
/* Enable GPIOB clock */
RCC_APB2PeriphClockCmd(LED_GPIO_CLK, ENABLE);
/* Configure LED pin as output push-pull */
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(LED_GPIO, &GPIO_InitStructure);
}
void TIM_Configuration(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
/* TIM3 clock enable */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE);
/* Time base configuration */
TIM_TimeBaseStructure.TIM_Period = 65535;
TIM_TimeBaseStructure.TIM_Prescaler = 7199;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure);
/* TIM3 enable counter */
TIM_Cmd(TIM3, ENABLE);
/* Enable the TIM3 global Interrupt */
NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
```
这里,我们使用了一个定时器来实现LED灯的闪烁。我们使用了TIM3定时器,将其时钟分频器设置为7200,这样它将每秒递增10次。我们还需要初始化中断向量表,以便在定时器计数到一定值时触发中断。
现在,我们需要编写中断处理程序:
```c
void TIM3_IRQHandler(void)
{
if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET)
{
if (led_on)
{
GPIO_ResetBits(LED_GPIO, LED_PIN);
led_on = 0;
}
else
{
GPIO_SetBits(LED_GPIO, LED_PIN);
led_on = 1;
}
TIM_ClearITPendingBit(TIM3, TIM_IT_Update);
}
}
```
在这里,我们检查定时器是否触发了更新中断,并根据LED灯的当前状态切换它的状态。
最后,我们需要编写主函数:
```c
int main(void)
{
GPIO_Configuration();
TIM_Configuration();
while (1)
{
if (GPIO_ReadInputDataBit(BUTTON_GPIO, BUTTON_PIN) == RESET && button_debounce == 0)
{
button_pressed = 1;
button_debounce = 1;
}
else if (GPIO_ReadInputDataBit(BUTTON_GPIO, BUTTON_PIN) == SET)
{
button_debounce = 0;
}
if (button_pressed && led_on)
{
TIM_ITConfig(TIM3, TIM_IT_Update, DISABLE);
button_pressed = 0;
GPIO_ResetBits(LED_GPIO, LED_PIN);
led_on = 0;
}
else if (button_pressed)
{
TIM_ITConfig(TIM3, TIM_IT_Update, ENABLE);
button_pressed = 0;
}
}
}
```
在主函数中,我们首先初始化GPIO和定时器。然后,我们进入一个循环,在循环中检查按钮是否按下,并在LED灯闪烁时停止它。如果按钮被按下并且LED灯正在闪烁,我们将禁用定时器并关闭LED灯。否则,我们将启用定时器,使LED灯开始闪烁。
这就是一个简单的STM32F103C6T6小游戏程序示例。请注意,此程序可能需要进行修改才能适应您的具体应用场景,因此请仔细阅读和理解代码,并进行必要的修改。
相关推荐
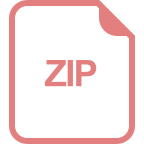














