c++编写程序,删除字符串中的某个字符。(字符串、要删除的字符都由用户从键盘输入,有则删除——所谓删除就是后序的元素往前覆盖,无则提示“该字符不存在。”) 例如: 输入原始字符串:abcebacebaghbf 输入要删除的字符: b 删除以后的字符串:aceaceaghf
时间: 2024-02-19 14:57:30 浏览: 104
好的,这是一个简单的字符串操作问题,可以用C++的string类来实现。以下是示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str, removeStr;
cout << "请输入原始字符串:";
getline(cin, str);
cout << "请输入要删除的字符:";
getline(cin, removeStr);
int pos = str.find(removeStr);
if (pos == string::npos) { // 该字符不存在
cout << "该字符不存在。" << endl;
} else {
str.erase(pos, removeStr.length()); // 从pos位置开始删除removeStr.length()个字符
cout << "删除以后的字符串:" << str << endl;
}
return 0;
}
```
在上面的代码中,我们首先使用 `getline()` 函数从标准输入中读取用户输入的字符串和要删除的字符,然后使用 `string` 类的 `find()` 函数查找要删除的字符在原始字符串中的位置,如果该字符不存在,则输出提示信息;否则使用 `erase()` 函数删除该字符。最后输出删除后的字符串即可。
相关问题
编写一个函数实现功能:从字符串中删除指定的字符。同一字母的大、小写按不同字符处理。例如:程序执行时输入字符串:turbo c and borland c++,从键盘输入字符n,则输出后变为:turbo c ad borlad c++。如果输入的字符在字符串中不存在,则字符串原样输出。
以下是Python代码实现:
def delete_char(string, char):
new_string = ""
for s in string:
if s != char and s != char.upper() and s != char.lower():
new_string += s
return new_string
string = input("请输入字符串:")
char = input("请输入要删除的字符:")
new_string = delete_char(string, char)
print("删除后的字符串为:", new_string)
c++删除输入字符串的某个字符
可以使用C++中的erase函数来删除字符串中的某个字符。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "hello";
char c = 'l';
size_t pos = str.find(c);
if (pos != std::string::npos) {
str.erase(pos, 1);
}
std::cout << str << std::endl; // 输出 "heo"
return 0;
}
```
在这个示例中,我们定义了一个字符串`str`和一个要删除的字符`c`。然后我们使用`find`函数来查找`c`在`str`中的位置。如果`c`存在,`find`函数返回其在字符串中的位置,我们使用`erase`函数删除该字符。最后,我们输出修改后的字符串`str`。
需要注意的是,`erase`函数的第一个参数是要删除字符的位置,第二个参数是要删除的字符数。在本例中,我们只删除一个字符,因此第二个参数为1。
阅读全文
相关推荐
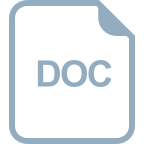
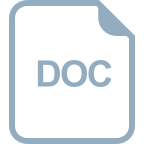
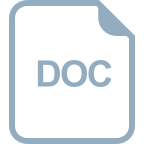












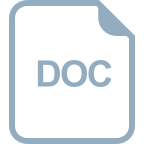
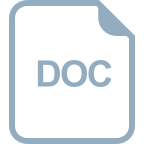
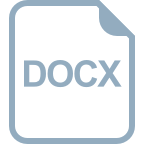