写一个获取中文首字母的js函数
时间: 2024-05-06 19:15:34 浏览: 137
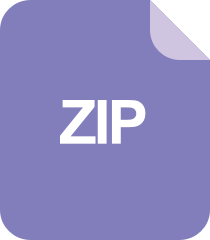
SortSelect vue + elementui自定义按首字母排序下拉列表组件
以下是一个获取中文首字母的 JavaScript 函数:
```javascript
function getFirstLetter(str) {
let firstLetter = "";
for (let i = 0; i < str.length; i++) {
const code = str.charCodeAt(i);
if (code >= 0x4e00 && code <= 0x9fa5) {
firstLetter += String.fromCharCode(((code - 0x4e00) / 588) + 0x41);
} else {
firstLetter += str[i];
}
}
return firstLetter;
}
```
这个函数接受一个字符串作为参数,遍历字符串中的每一个字符,如果该字符是汉字,则根据汉字的 Unicode 编码计算出其对应的拼音首字母,并将其添加到返回值中;如果该字符不是汉字,则直接将其添加到返回值中。最终返回的字符串即为输入字符串的拼音首字母。
阅读全文
相关推荐
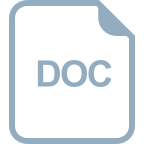
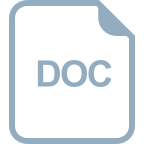

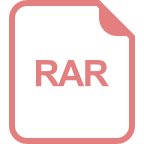
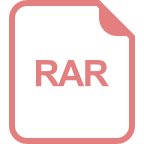
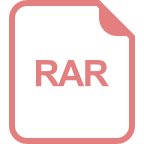
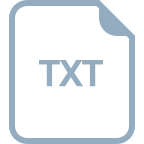


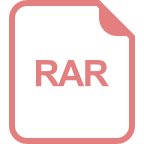
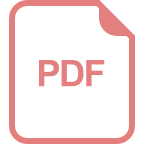
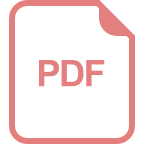


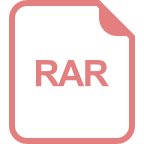
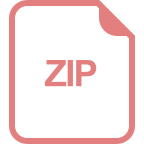
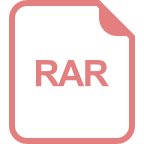
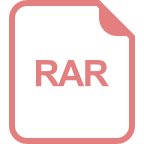