openlayers 点位添加点击事件
时间: 2023-10-01 12:03:50 浏览: 34
好的,关于openlayers点位添加点击事件的问题,我可以为您解答。您可以通过以下代码来添加点击事件:
```javascript
var marker = new ol.Feature({
geometry: new ol.geom.Point(ol.proj.fromLonLat([lon, lat])),
name: 'Marker ' + i
});
// 添加点击事件
marker.on('click', function(event){
console.log('您点击了Marker:' + marker.getProperties().name);
});
var vectorSource = new ol.source.Vector({
features: [marker]
});
var vectorLayer = new ol.layer.Vector({
source: vectorSource
});
map.addLayer(vectorLayer);
```
其中,`marker`为添加的点位,`lon`和`lat`为点位的经纬度坐标,在`on`方法中绑定了点击事件,并在点击事件中获取了点位的名称。
相关问题
vue 中 openlayers 点位添加点击事件
很高兴为您解答问题。对于Vue中OpenLayers点位添加点击事件,你可以这样来实现:
1. 在Vue组件中引入OpenLayers,比如:
import Map from 'ol/Map';
import View from 'ol/View';
import { fromLonLat } from 'ol/proj';
import TileLayer from 'ol/layer/Tile';
import XYZ from 'ol/source/XYZ';
import Feature from 'ol/Feature';
import Point from 'ol/geom/Point';
import VectorLayer from 'ol/layer/Vector';
import VectorSource from 'ol/source/Vector';
import { Style, Icon } from 'ol/style';
2. 在Vue组件中创建map对象并给定初始的坐标和缩放等级,比如:
created() {
this.map = new Map({
target: 'map',
layers: [
new TileLayer({
source: new XYZ({
url: 'https://{a-c}.tile.openstreetmap.org/{z}/{x}/{y}.png'
})
})
],
view: new View({
center: fromLonLat([104, 30]),
zoom: 13
})
});
}
3. 创建一个VectorSource和VectorLayer用于添加点位,比如:
this.source = new VectorSource();
this.layer = new VectorLayer({
source: this.source
});
this.map.addLayer(this.layer);
4. 创建一个Feature并给定点位的坐标和样式,比如:
const feature = new Feature({
geometry: new Point(fromLonLat([104.0668, 30.5728])),
name: '测试点位',
type: 'test',
population: 4000,
rainfall: 500
});
const iconStyle = new Style({
image: new Icon({
src: '/assets/icon.png',
scale: 0.8,
anchor: [0.5, 1]
})
});
feature.setStyle(iconStyle);
5. 给创建的Feature添加click事件,并在事件中实现点击后的操作,比如:
feature.on('click', (event) => {
console.log('点击了点位:', event.target.values_.name);
alert('点击了点位:' + event.target.values_.name);
});
6. 最后将创建的Feature添加到VectorSource中,比如:
this.source.addFeature(feature);
以上就是Vue中OpenLayers点位添加点击事件的实现方法,希望能对你有所帮助。
vue openlayers 聚合点位点击事件
要实现在 Vue 中使用 OpenLayers 实现聚合点位点击事件,可以按照以下步骤进行:
1. 在 Vue 中安装 OpenLayers:可以使用命令 `npm install ol` 安装 OpenLayers。
2. 在 Vue 中创建地图容器:可以在 Vue 的 `template` 中创建一个 `div` 容器作为地图容器。
3. 在 Vue 中使用 OpenLayers 创建地图:可以在 Vue 的 `methods` 中创建一个函数,使用 OpenLayers 创建地图。
4. 在地图中添加聚合点位图层:在创建地图的过程中,可以添加一个聚合点位图层。
5. 监听聚合点位图层的点击事件:可以在创建地图的过程中,使用 OpenLayers 提供的 `on` 方法监听聚合点位图层的点击事件。
6. 在点击事件中获取聚合点位的信息:在监听到聚合点位图层的点击事件后,可以获取聚合点位的信息并进行相应处理。
以下是一个基本的 Vue 组件代码示例,用于实现 OpenLayers 中聚合点位的点击事件:
```html
<template>
<div id="map"></div>
</template>
<script>
import 'ol/ol.css';
import { Map, View } from 'ol';
import { fromLonLat } from 'ol/proj';
import { Cluster, OSM, Vector } from 'ol/source';
import { Tile as TileLayer, Vector as VectorLayer } from 'ol/layer';
import Feature from 'ol/Feature';
import Point from 'ol/geom/Point';
export default {
name: 'Map',
mounted() {
this.initMap();
},
methods: {
initMap() {
const source = new Cluster({
distance: 40,
source: new Vector({
url: 'data/kml/2012_Earthquakes_Mag5.kml',
format: new KML({
extractStyles: false,
}),
}),
});
const clusterLayer = new VectorLayer({
source: source,
});
const map = new Map({
target: 'map',
layers: [
new TileLayer({
source: new OSM(),
}),
clusterLayer,
],
view: new View({
center: fromLonLat([-117.1497, 32.6965]),
zoom: 12,
}),
});
clusterLayer.getSource().on('addfeature', function (event) {
const cluster = event.feature;
if (cluster.get('features').length > 1) {
const coordinates = cluster.getGeometry().getCoordinates();
const feature = new Feature(new Point(coordinates));
feature.set('cluster', cluster);
source.addFeature(feature);
}
});
clusterLayer.getSource().on('click', function (event) {
const feature = map.forEachFeatureAtPixel(event.pixel, function (feature) {
return feature;
});
if (feature && feature.get('cluster')) {
const cluster = feature.get('cluster');
const features = cluster.get('features');
const coordinate = cluster.getGeometry().getCoordinates();
console.log(features);
console.log(coordinate);
}
});
},
},
};
</script>
<style>
#map {
height: 400px;
}
</style>
```
在上述代码示例中,我们使用 OpenLayers 创建了一个聚合点位图层,并在地图中添加了该图层。然后,我们使用 `on` 方法来监听聚合点位图层的点击事件,并在点击事件中获取聚合点位的信息。
相关推荐
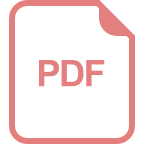












