用Java写一个俄罗斯方块小程序
时间: 2023-12-05 16:05:20 浏览: 109
好的,我可以为您提供一个简单的 Java 俄罗斯方块小程序的示例代码。这个程序基于 Java Swing 库,使用了 JFrame、JPanel、JLabel、JButton 等组件,实现了一个简单的俄罗斯方块游戏。
以下是示例代码,您可以将其保存为 Tetris.java 并运行:
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class Tetris extends JPanel implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 300;
private static final int HEIGHT = 600;
private static final int BLOCK_SIZE = 30;
private static final int BOARD_WIDTH = 10;
private static final int BOARD_HEIGHT = 20;
private static final int DELAY = 500;
private static final Color[] COLORS = { Color.RED, Color.GREEN, Color.BLUE, Color.YELLOW, Color.PINK, Color.ORANGE,
Color.CYAN };
private JFrame frame;
private JPanel board;
private JLabel scoreLabel;
private JButton startButton;
private int[][] blocks;
private Point[] currentBlock;
private Color currentColor;
private int currentX;
private int currentY;
private boolean isRunning;
private int score;
public Tetris() {
frame = new JFrame("Tetris");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
board = new JPanel() {
private static final long serialVersionUID = 1L;
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (int i = 0; i < BOARD_HEIGHT; i++) {
for (int j = 0; j < BOARD_WIDTH; j++) {
if (blocks[i][j] != 0) {
g.setColor(COLORS[blocks[i][j] - 1]);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
for (Point p : currentBlock) {
g.setColor(currentColor);
g.fillRect((currentX + p.x) * BLOCK_SIZE, (currentY + p.y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
};
board.setPreferredSize(new Dimension(BLOCK_SIZE * BOARD_WIDTH, BLOCK_SIZE * BOARD_HEIGHT));
frame.add(board, BorderLayout.CENTER);
JPanel bottomPanel = new JPanel();
scoreLabel = new JLabel("Score: 0");
bottomPanel.add(scoreLabel);
startButton = new JButton("Start");
startButton.addActionListener(this);
bottomPanel.add(startButton);
frame.add(bottomPanel, BorderLayout.SOUTH);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
private void init() {
blocks = new int[BOARD_HEIGHT][BOARD_WIDTH];
currentBlock = new Point[4];
generateBlock();
currentX = BOARD_WIDTH / 2 - 1;
currentY = 0;
isRunning = true;
score = 0;
scoreLabel.setText("Score: " + score);
}
private void generateBlock() {
Random rand = new Random();
int type = rand.nextInt(7) + 1;
switch (type) {
case 1: // I
currentBlock[0] = new Point(0, 0);
currentBlock[1] = new Point(0, 1);
currentBlock[2] = new Point(0, 2);
currentBlock[3] = new Point(0, 3);
break;
case 2: // J
currentBlock[0] = new Point(0, 0);
currentBlock[1] = new Point(1, 0);
currentBlock[2] = new Point(1, 1);
currentBlock[3] = new Point(1, 2);
break;
case 3: // L
currentBlock[0] = new Point(0, 2);
currentBlock[1] = new Point(1, 0);
currentBlock[2] = new Point(1, 1);
currentBlock[3] = new Point(1, 2);
break;
case 4: // O
currentBlock[0] = new Point(0, 0);
currentBlock[1] = new Point(0, 1);
currentBlock[2] = new Point(1, 0);
currentBlock[3] = new Point(1, 1);
break;
case 5: // S
currentBlock[0] = new Point(0, 1);
currentBlock[1] = new Point(0, 2);
currentBlock[2] = new Point(1, 0);
currentBlock[3] = new Point(1, 1);
break;
case 6: // T
currentBlock[0] = new Point(0, 1);
currentBlock[1] = new Point(1, 0);
currentBlock[2] = new Point(1, 1);
currentBlock[3] = new Point(1, 2);
break;
case 7: // Z
currentBlock[0] = new Point(0, 0);
currentBlock[1] = new Point(0, 1);
currentBlock[2] = new Point(1, 1);
currentBlock[3] = new Point(1, 2);
break;
}
currentColor = COLORS[rand.nextInt(COLORS.length)];
}
private void moveDown() {
if (canMove(currentX, currentY + 1, currentBlock)) {
currentY++;
} else {
addBlock(currentX, currentY, currentBlock, currentColor);
checkRows();
generateBlock();
currentX = BOARD_WIDTH / 2 - 1;
currentY = 0;
if (!canMove(currentX, currentY, currentBlock)) {
isRunning = false;
}
}
}
private void moveLeft() {
if (canMove(currentX - 1, currentY, currentBlock)) {
currentX--;
}
}
private void moveRight() {
if (canMove(currentX + 1, currentY, currentBlock)) {
currentX++;
}
}
private void rotate() {
Point[] newBlock = new Point[4];
for (int i = 0; i < 4; i++) {
newBlock[i] = new Point(currentBlock[i].y, -currentBlock[i].x);
}
if (canMove(currentX, currentY, newBlock)) {
currentBlock = newBlock;
}
}
private boolean canMove(int x, int y, Point[] block) {
for (Point p : block) {
int newX = x + p.x;
int newY = y + p.y;
if (newX < 0 || newX >= BOARD_WIDTH || newY < 0 || newY >= BOARD_HEIGHT || blocks[newY][newX] != 0) {
return false;
}
}
return true;
}
private void addBlock(int x, int y, Point[] block, Color color) {
for (Point p : block) {
blocks[y + p.y][x + p.x] = COLORS.length + 1;
}
for (Point p : block) {
blocks[y + p.y][x + p.x] = COLORS.length + 1 - colorIndex(color);
}
}
private int colorIndex(Color color) {
for (int i = 0; i < COLORS.length; i++) {
if (color.equals(COLORS[i])) {
return i;
}
}
return -1;
}
private void checkRows() {
ArrayList<Integer> fullRows = new ArrayList<Integer>();
for (int i = 0; i < BOARD_HEIGHT; i++) {
boolean full = true;
for (int j = 0; j < BOARD_WIDTH; j++) {
if (blocks[i][j] == 0) {
full = false;
break;
}
}
if (full) {
fullRows.add(i);
}
}
if (fullRows.size() > 0) {
for (int i : fullRows) {
for (int j = i; j > 0; j--) {
for (int k = 0; k < BOARD_WIDTH; k++) {
blocks[j][k] = blocks[j - 1][k];
}
}
}
score += fullRows.size() * 100;
scoreLabel.setText("Score: " + score);
}
}
private void gameLoop() {
while (isRunning) {
moveDown();
board.repaint();
try {
Thread.sleep(DELAY);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
init();
gameLoop();
}
}
public static void main(String[] args) {
new Tetris();
}
}
```
该程序使用一个二维数组来表示游戏区域,每个格子可以被染上不同的颜色。在游戏中,程序会随机生成一个俄罗斯方块,玩家需要使用键盘控制方块的移动和旋转,让方块落到最底部或者尽可能占据更多的空间。当一行满了格子时,该行会被消除,并且玩家可以获得一定的分数。当方块无法继续下落时,游戏结束。
阅读全文
相关推荐
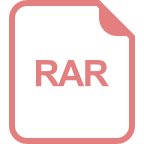
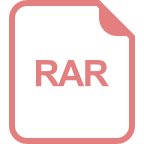
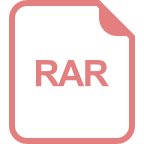
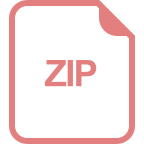
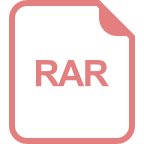


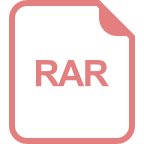

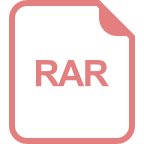
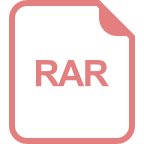
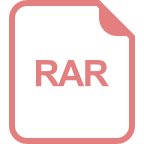



