flask登录注册页面
时间: 2023-07-01 12:18:04 浏览: 83
好的,下面是一个简单的 Flask 登录注册页面的示例代码,包括了表单提交、数据验证、密码加密等功能:
```
from flask import Flask, render_template, request, redirect, url_for, flash
from flask_sqlalchemy import SQLAlchemy
from werkzeug.security import generate_password_hash, check_password_hash
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key_here'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(50), unique=True, nullable=False)
password = db.Column(db.String(100), nullable=False)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
confirm_password = request.form['confirm_password']
if not username or not password or not confirm_password:
flash('Please fill in all the fields.')
return redirect(url_for('register'))
if password != confirm_password:
flash('Passwords do not match.')
return redirect(url_for('register'))
if User.query.filter_by(username=username).first():
flash('Username already exists.')
return redirect(url_for('register'))
hashed_password = generate_password_hash(password)
new_user = User(username=username, password=hashed_password)
db.session.add(new_user)
db.session.commit()
flash('You have successfully registered.')
return redirect(url_for('login'))
return render_template('register.html')
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
if not username or not password:
flash('Please fill in all the fields.')
return redirect(url_for('login'))
user = User.query.filter_by(username=username).first()
if not user or not check_password_hash(user.password, password):
flash('Username or password is incorrect.')
return redirect(url_for('login'))
flash('You have successfully logged in.')
return redirect(url_for('index'))
return render_template('login.html')
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
```
其中,`index.html`、`register.html` 和 `login.html` 分别是主页、注册页面和登录页面的 HTML 模板文件,可以根据自己的需要进行修改。同时,需要注意引入的 Flask 扩展包和模块,以及数据库模型的定义和数据库的初始化等细节问题。
相关推荐
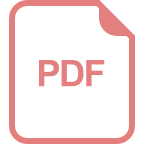













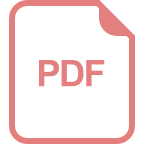