python批量搜索微信好友
时间: 2023-08-12 19:03:34 浏览: 261
可以使用itchat库来实现Python批量搜索微信好友。首先需要安装itchat库,然后编写以下代码:
```python
import itchat
itchat.auto_login()
friends = itchat.search_friends(name='好友昵称')
for friend in friends:
print(friend['NickName'], friend['RemarkName'], friend['UserName'])
```
其中,`name`参数为好友的昵称,可以通过昵称进行搜索。执行以上代码后,会输出好友的昵称、备注和用户名。可以根据需要对搜索结果进行处理。
相关问题
python批量添加微信好友
批量添加微信好友通常是通过Python脚本配合第三方库,如WeChat API或者微信网页版的自动化操作来实现的,但这需要满足几个条件:
1. **合法授权**:你需要有微信的开发者账号,并获得相应的API权限,通常用于管理企业号或者小程序,而不是个人用户。
2. **好友请求自动化**:一些工具可能会模拟点击“添加到通讯录”按钮,但请注意这涉及到微信的反爬虫策略,长期大量操作可能会导致封号风险。
3. **好友列表获取**:你需要获取到对方的微信ID(微信号或手机号),这个过程可能涉及到隐私问题,需要得到对方的明确同意。
下面是一个简化版的概念示例,实际应用中会更复杂并需要处理登录、授权等步骤:
```python
import wechat_api # 假设存在一个模拟微信API的库
def add_friends(user_id_list):
client = wechat_api.login() # 登录微信
for user_id in user_id_list:
response = client.add_friend(user_id) # 添加好友请求
if response.status == 'success':
print(f"已向{user_id}发送好友请求")
else:
print(f"添加{user_id}失败,错误信息:{response.error_message}")
# 使用你从其他途径获取的用户ID列表
friend_ids_to_add = ['your_friend_id1', 'your_friend_id2']
add_friends(friend_ids_to_add)
```
python通过手机号批量搜索微信好友并保存头像到文件夹
要通过手机号批量搜索微信好友并保存头像到文件夹,可以使用itchat库来实现。首先需要安装itchat库,然后编写以下代码:
```python
import itchat
import os
# 登录微信
itchat.auto_login()
# 手机号列表,用于搜索微信好友
phone_list = ['手机号1', '手机号2', '手机号3']
# 循环搜索好友
for phone in phone_list:
friends = itchat.search_friends(phone=phone)
for friend in friends:
# 保存好友头像到文件夹
img = itchat.get_head_img(userName=friend['UserName'])
img_path = os.path.join('头像文件夹', f"{friend['NickName']}.jpg")
with open(img_path, 'wb') as f:
f.write(img)
# 退出微信登录
itchat.logout()
```
其中,`phone_list`参数为手机号列表,用于搜索微信好友。执行以上代码后,会循环搜索每个手机号对应的微信好友,并保存好友的头像到指定的文件夹中。可以根据需要对搜索结果进行处理。注意,如果要使用该功能,需要先获取好友的手机号,并在好友的个人信息中填写手机号。
阅读全文
相关推荐
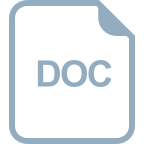
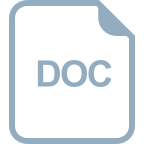
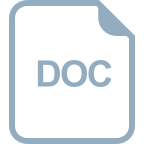
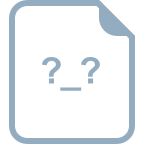
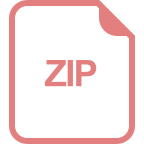

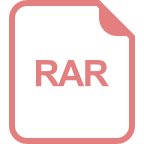
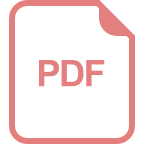
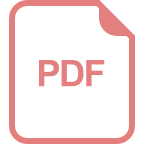
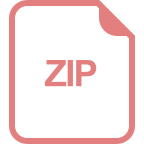
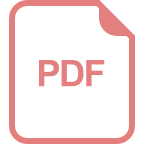
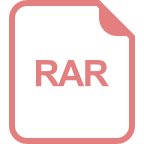
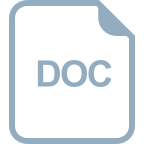


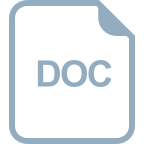
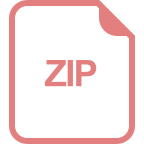
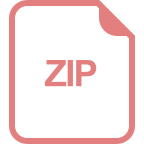