将swapf aux,f转换成C语言
时间: 2023-08-16 13:04:21 浏览: 173
在C语言中,变量的交换可以通过一个临时变量来实现。因此,将swapf aux,f转换成C语言可以写为:
```c
unsigned char temp = aux;
aux = f;
f = temp;
```
这段代码的意思是,将变量aux的值存储到临时变量temp中,然后将变量f的值赋给变量aux,最后将临时变量temp中存储的值赋给变量f,完成变量的交换。
相关问题
编程题(函数模板)。编写一个函数模板swapf,基本原型为: void swap( T &x, T &y);功能为交换2个参数x和y的值。然后编写主程序,分别交换整数、浮点数和字符串数据。
函数模板是一种通用的设计模式,在C++中允许程序员编写一次函数定义,然后可以在多种数据类型上应用。`swapf`函数模板的基本思想就是利用C++的模板特性,将类型的不确定性传递给编译器处理。以下是`swapf`函数模板的实现以及如何在主程序中使用它来交换不同类型的值(如整数(int)、浮点数(float)和字符串(string)`):
```cpp
// 定义swapf函数模板,接受任意类型T并引用两个变量x和y
template <typename T>
void swap(T& x, T& y) {
// 使用T的赋值运算符实现值的交换
T temp = x;
x = y;
y = temp;
}
int main() {
// 主程序部分,分别对不同类型的数据进行交换
int a = 5, b = 10;
swap(a, b);
std::cout << "After swapping integers: a = " << a << ", b = " << b << "\n";
float f1 = 3.14, f2 = 2.71;
swap(f1, f2);
std::cout << "After swapping floats: f1 = " << f1 << ", f2 = " << f2 << "\n";
std::string str1 = "Hello", str2 = "World";
swap(str1, str2);
std::cout << "After swapping strings: str1 = " << str1 << ", str2 = " << str2 << "\n";
return 0;
}
```
在这个例子中,当我们调用`swap(a, b)`、`swap(f1, f2)`和`swap(str1, str2)`时,编译器会根据相应的类型生成特定版本的`swap`函数,并完成实际的值交换。
编程题(函数模板)。编写一个函数模板swapf,基本原型为: void swap( T &x, T &y);功能为交换2个参数x和y的值。然后编写主程序,分别交换整数、浮点数和字符串数据。
在编程中,函数模板是一种通用的设计技巧,允许你在编写一次函数定义的情况下处理多种数据类型。这里有一个关于如何创建一个名为`swapf`的函数模板示例,它接受两个`T`类型的引用并交换它们的值。`T`是一个类型参数,代表任何可以复制的基本数据类型,如int、float或char*(对于字符串)。
```cpp
template <typename T>
void swap(T& x, T& y) {
T temp = x;
x = y;
y = temp;
}
// 主程序演示如何使用这个模板
int main() {
int a = 5, b = 10;
float c = 3.14, d = 2.718;
std::string str1 = "Hello", str2 = "World";
// 交换整数
swap(a, b);
std::cout << "After swapping integers: a=" << a << ", b=" << b << std::endl;
// 交换浮点数
swap(c, d);
std::cout << "After swapping floats: c=" << c << ", d=" << d << std::endl;
// 交换字符串
swap(str1, str2);
std::cout << "After swapping strings: str1=\"" << str1 << "\", str2=\"" << str2 << "\"\n";
return 0;
}
```
在这个例子中,`swap`函数模板可以根据传入的具体类型自动调整操作。在main函数中,我们展示了如何使用`swapf`函数分别交换整数、浮点数和字符串。
阅读全文
相关推荐












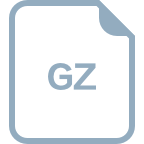

