用python写一个爬虫小程序
时间: 2024-05-29 19:03:52 浏览: 144
好的,我可以为你写一个 Python 爬虫小程序的示例。
首先,我们需要安装一些库,包括 `requests` 和 `BeautifulSoup`。你可以使用以下命令进行安装:
```
pip install requests
pip install beautifulsoup4
```
然后,我们可以使用 `requests` 库发起一个 HTTP 请求来获取网页的源代码。例如,我们可以使用以下代码来获取百度首页的源代码:
```python
import requests
url = "http://www.baidu.com"
response = requests.get(url)
html = response.text
print(html)
```
接下来,我们可以使用 `BeautifulSoup` 库来解析网页源代码,并提取我们感兴趣的信息。例如,我们可以使用以下代码来提取百度首页上的所有链接:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, "html.parser")
links = soup.find_all("a")
for link in links:
print(link.get("href"))
```
最后,我们可以将这些代码结合起来,并加上一些循环和判断逻辑,就可以写出一个简单的爬虫程序了。例如,我们可以写一个爬虫程序来抓取百度首页上的所有链接,并递归地访问这些链接所对应的页面,直到所有页面都被访问过为止。
希望这个示例能帮到你
相关问题
麻烦用python程序写一个爬虫小案例,使用场景输入手机号 获取验证码
当然可以,以下是一个简单的 Python 爬虫小案例,可以用于输入手机号获取验证码:
```python
import requests
phone_number = input("请输入手机号:")
# 发送请求获取验证码
response = requests.get(f"https://example.com/api/get_code?phone={phone_number}")
# 解析响应内容,获取验证码
code = response.json()["code"]
print(f"验证码为:{code}")
```
请注意,这只是一个简单的示例,实际情况可能会更加复杂,需要根据具体场景进行调整。同时,也要注意遵守相关法律法规,不要进行违法操作。
python爬虫小程序案例
你好,以下是一个 Python 爬虫小程序案例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 获取页面标题
title = soup.title.string
print('页面标题:', title)
# 获取页面所有链接
links = []
for link in soup.find_all('a'):
links.append(link.get('href'))
print('页面链接:', links)
```
希望对你有帮助!
阅读全文
相关推荐
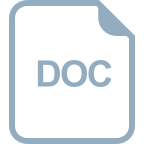
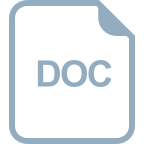
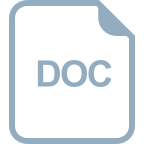
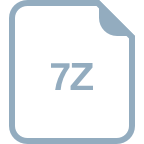
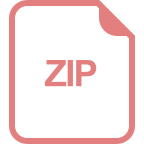
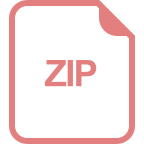
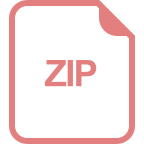
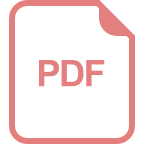
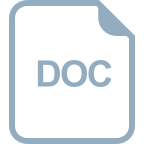
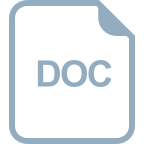
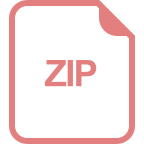
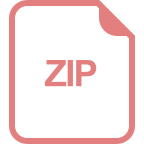
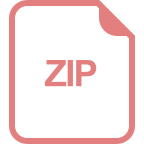
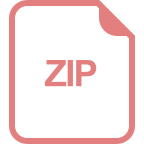
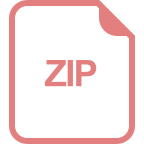