sem_t转int型
时间: 2023-07-12 13:11:14 浏览: 64
sem_t类型表示一个信号量,它是一个结构体,无法直接转换为int类型。如果需要将sem_t类型转换为int类型,可以使用sem_getvalue函数获取信号量的值,该函数将信号量的值存储在一个int类型的变量中。例如:
```
#include <semaphore.h>
sem_t my_sem;
int sem_value;
// 初始化信号量
sem_init(&my_sem, 0, 1);
// 获取信号量的值
sem_getvalue(&my_sem, &sem_value);
// 将信号量的值转换为int类型
int sem_int = (int)sem_value;
```
相关问题
sem_init的使用
sem_init 是一个用于初始化信号量的函数。它的型如下:
```c
int sem_init(sem_t *sem, int pshared, unsigned int value);
```
其中,`sem` 是指向信号量对象的指针,`pshared` 用于指定是否在进程间共享信号量,`value` 是信号量的初始值。
调用 `sem_init` 函数可以创建一个新的信号量,并初始化它的值。如果 `pshared` 参数为非零值,表示该信号量将在进程间共享,否则只在当前进程中使用。`value` 参数指定了信号量的初始值。
例如,下面的代码片段展示了如何使用 `sem_init` 初始化一个信号量:
```c
#include <stdio.h>
#include <semaphore.h>
int main() {
sem_t my_sem;
int ret = sem_init(&my_sem, 0, 1);
if (ret == -1) {
perror("Semaphore initialization failed");
return 1;
}
// 使用信号量进行同步操作...
return 0;
}
```
在这个示例中,我们创建了一个名为 `my_sem` 的信号量,并将其初始化为 1。这意味着初始时信号量的值为 1,可以用于实现互斥操作或者限制并发访问资源。
编写一个程序,POSIX信号量,实现如下功能:包含一个生产者线程和一个消费者线程,它们有一个共享缓冲区;共享缓冲区是一个长度为10的字符型数组,该数组为全局变量;生产者从键盘获取一个用户输入的字符串(长度不超过255字符),然后将字符串逐字符地存入共享缓冲区;消费者从共享缓冲区中逐字符地读取并打印字符;当检测到输入字符串中的‘!’时,退出程序。使用sem_init、sem_wait、sem_post、sem_destroy等函数
好的,以下是实现该功能的代码,注意需要在 Linux 环境下编译:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <string.h>
#define BUFFER_SIZE 10
char buffer[BUFFER_SIZE]; // 共享缓冲区
sem_t empty, full; // 信号量
void *producer(void *arg) {
while (1) {
char input[256];
printf("请输入字符串:");
fgets(input, 256, stdin);
input[strlen(input) - 1] = '\0'; // 去掉换行符
int len = strlen(input);
for (int i = 0; i < len; i++) {
sem_wait(&empty); // 等待空缓冲区
buffer[i % BUFFER_SIZE] = input[i];
sem_post(&full); // 增加字符数量
if (input[i] == '!') {
return NULL; // 检测到退出字符
}
}
}
}
void *consumer(void *arg) {
while (1) {
sem_wait(&full); // 等待非空缓冲区
char ch = buffer[strlen(buffer) - sem_getvalue(&full, NULL) - 1];
printf("%c", ch);
sem_post(&empty); // 增加空缓冲区数量
if (ch == '!') {
return NULL; // 检测到退出字符
}
}
}
int main() {
pthread_t t_producer, t_consumer;
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
pthread_create(&t_producer, NULL, producer, NULL);
pthread_create(&t_consumer, NULL, consumer, NULL);
pthread_join(t_producer, NULL);
pthread_join(t_consumer, NULL);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
```
在程序中,我们定义了两个线程,一个是生产者线程,一个是消费者线程。生产者从键盘获取用户输入的字符串,并逐字符地存入共享缓冲区,消费者从共享缓冲区中逐字符地读取并打印字符。当检测到输入字符串中的 '!' 时,退出程序。同时,我们使用了信号量来保证生产者和消费者之间的同步和互斥。
在 `main` 函数中,我们调用了 `pthread_create` 函数创建生产者和消费者线程,并调用 `pthread_join` 函数等待线程结束。我们还使用了 `sem_init` 函数初始化信号量,`sem_wait` 函数等待信号量,`sem_post` 函数增加信号量,`sem_destroy` 函数销毁信号量。
相关推荐
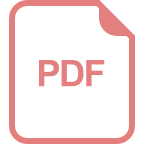












