用C++编一个模拟比特币挖掘界面
时间: 2024-06-08 11:10:38 浏览: 139
好的,以下是一个简单的模拟比特币挖掘界面的C++代码:
```c++
#include <iostream>
#include <string>
#include <ctime>
#include <cstdlib>
using namespace std;
// 定义区块结构体
struct Block {
int index;
string data;
string timestamp;
string previousHash;
string hash;
int nonce;
};
// 计算哈希值函数
string calculateHash(int index, string data, string timestamp, string previousHash, int nonce) {
string str = to_string(index) + data + timestamp + previousHash + to_string(nonce);
return str; // 这里简化了哈希计算过程,直接将所有信息拼接起来返回
}
// 随机数生成函数
int generateRandomNumber() {
return rand();
}
// 挖矿函数
Block mineBlock(int index, string data, string previousHash) {
string timestamp = to_string(time(NULL));
int nonce = 0;
string hash = "";
while (hash.substr(0, 4) != "0000") { // 满足哈希前4位是0的条件
nonce = generateRandomNumber();
hash = calculateHash(index, data, timestamp, previousHash, nonce);
}
Block block = {index, data, timestamp, previousHash, hash, nonce};
return block;
}
// 主函数
int main() {
srand(time(NULL)); // 初始化随机数生成器
int numBlocks;
cout << "请输入要生成的区块数量:";
cin >> numBlocks;
cout << endl;
// 生成创世区块
Block genesisBlock = {0, "Genesis Block", to_string(time(NULL)), "0", "", 0};
genesisBlock.hash = calculateHash(genesisBlock.index, genesisBlock.data, genesisBlock.timestamp, genesisBlock.previousHash, genesisBlock.nonce);
// 打印创世区块信息
cout << "区块" << genesisBlock.index << ":\n";
cout << "数据:" << genesisBlock.data << endl;
cout << "时间戳:" << genesisBlock.timestamp << endl;
cout << "前一区块哈希值:" << genesisBlock.previousHash << endl;
cout << "当前哈希值:" << genesisBlock.hash << endl;
cout << "随机数:" << genesisBlock.nonce << endl;
cout << endl;
// 生成其他区块
Block previousBlock = genesisBlock;
for (int i = 1; i < numBlocks; i++) {
Block block = mineBlock(i, "Block " + to_string(i), previousBlock.hash);
previousBlock = block;
// 打印区块信息
cout << "区块" << block.index << ":\n";
cout << "数据:" << block.data << endl;
cout << "时间戳:" << block.timestamp << endl;
cout << "前一区块哈希值:" << block.previousHash << endl;
cout << "当前哈希值:" << block.hash << endl;
cout << "随机数:" << block.nonce << endl;
cout << endl;
}
return 0;
}
```
运行程序后,会提示输入要生成的区块数量。程序会先生成创世区块,然后根据前一个区块的哈希值生成后续区块,直到达到指定数量。每个区块的哈希值需要满足前4位是0的条件,否则就需要重新生成随机数。程序会打印每个区块的信息,包括数据、时间戳、前一区块哈希值、当前哈希值和随机数。
相关推荐
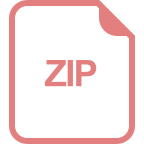
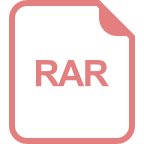
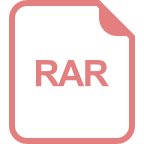
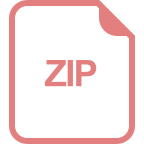
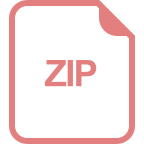
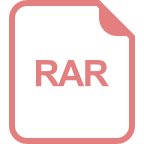
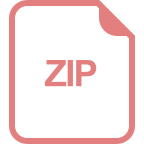
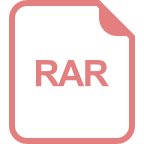
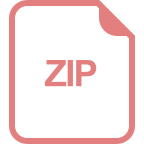
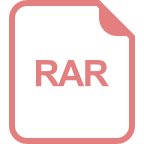
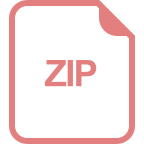