C# 实现多线程步骤编辑器
时间: 2023-06-29 10:01:54 浏览: 59
实现多线程步骤编辑器的步骤如下:
1. 创建一个窗体应用程序或控制台应用程序。
2. 创建一个类来表示每个步骤,包括步骤名称、描述和执行的代码。
3. 创建一个数据结构来保存步骤列表。
4. 在主线程中创建一个 GUI,用于显示步骤列表和当前步骤的详细信息。
5. 创建一个后台工作线程来执行步骤。
6. 在后台线程中循环遍历步骤列表,依次执行每个步骤。
7. 在主线程中使用委托将更新 GUI 的任务委托给主线程。
8. 在后台线程中使用线程同步机制来确保数据的正确性和同步性。
以下是一个简单的 C# 代码示例,展示了如何实现一个多线程步骤编辑器:
```csharp
using System;
using System.Threading;
using System.Windows.Forms;
namespace MultiThreadedStepEditor
{
public partial class MainForm : Form
{
private StepList _steps;
private Thread _workerThread;
private bool _isRunning;
public MainForm()
{
InitializeComponent();
_steps = new StepList();
_steps.Add(new Step("Step 1", "Description of step 1", () => { /* Code to execute for step 1 */ }));
_steps.Add(new Step("Step 2", "Description of step 2", () => { /* Code to execute for step 2 */ }));
_steps.Add(new Step("Step 3", "Description of step 3", () => { /* Code to execute for step 3 */ }));
_steps.Add(new Step("Step 4", "Description of step 4", () => { /* Code to execute for step 4 */ }));
}
private void MainForm_Load(object sender, EventArgs e)
{
UpdateStepList();
}
private void UpdateStepList()
{
lstSteps.Items.Clear();
foreach (var step in _steps)
{
lstSteps.Items.Add(step.Name);
}
}
private void btnRun_Click(object sender, EventArgs e)
{
if (_isRunning)
{
_workerThread.Abort();
_isRunning = false;
btnRun.Text = "Run";
return;
}
_isRunning = true;
btnRun.Text = "Stop";
_workerThread = new Thread(() =>
{
foreach (var step in _steps)
{
if (!_isRunning)
{
break;
}
// Execute the code for the current step
step.Action();
// Update the UI with the current step
this.Invoke(new Action(() =>
{
lblCurrentStep.Text = step.Name;
}));
}
// Reset the UI when the work is done
this.Invoke(new Action(() =>
{
lblCurrentStep.Text = "None";
btnRun.Text = "Run";
_isRunning = false;
}));
});
_workerThread.Start();
}
}
public class Step
{
public string Name { get; set; }
public string Description { get; set; }
public Action Action { get; set; }
public Step(string name, string description, Action action)
{
Name = name;
Description = description;
Action = action;
}
}
public class StepList : System.Collections.Generic.List<Step>
{
}
}
```
在该示例中,我们创建了一个 `Step` 类来表示每个步骤,然后创建了一个 `StepList` 类来保存步骤列表。在主窗口中,我们使用一个 `ListBox` 控件来显示步骤列表,并使用一个 `Label` 控件来显示当前步骤的详细信息。当用户单击“运行”按钮时,我们创建一个后台工作线程来执行步骤列表,并在界面上更新当前步骤。如果用户单击“停止”按钮,我们终止后台线程并重置界面状态。
相关推荐
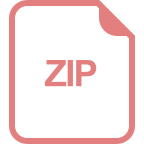
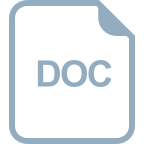
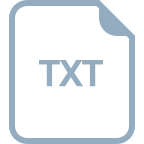














