vue3+ts监听滚动条触底事件
时间: 2023-08-30 09:05:17 浏览: 85
好的,关于vue3 ts监听滚动条触底事件,你可以使用IntersectionObserver API实现,代码实现可参考以下示例:
```
// 使用IntersectionObserver API监听滚动条触底事件
const observer = new IntersectionObserver(entries => {
// 如果目标元素相交,则执行相应操作
if (entries[0].isIntersecting) {
console.log("触底了!");
// 在这里执行你的代码,比如异步请求更多数据
}
});
// 获取要监听的元素
const target = document.querySelector("#target");
// 将目标元素添加到观察列表中
observer.observe(target);
```
相关问题
vue2+ts监听滚动条
在Vue2中,可以通过监听window的scroll事件来实现滚动条的滚动监听。具体步骤如下:
1. 在组件的created钩子函数中,使用window.addEventListener方法监听scroll事件,并绑定一个回调函数。
```typescript
created() {
window.addEventListener('scroll', this.handleScroll)
},
```
2. 在组件的destroyed钩子函数中,使用window.removeEventListener方法移除scroll事件的监听。
```typescript
destroyed() {
window.removeEventListener('scroll', this.handleScroll)
},
```
3. 在回调函数handleScroll中,获取滚动条的滚动距离,并根据业务需求进行处理。
```typescript
methods: {
handleScroll() {
const scrollTop = window.pageYOffset || document.documentElement.scrollTop || document.body.scrollTop
// 处理业务逻辑
}
}
```
完整代码如下:
```typescript
<template>
<div>
<!-- content -->
</div>
</template>
<script lang="ts">
import { Component, Vue } from 'vue-property-decorator'
@Component
export default class MyComponent extends Vue {
created() {
window.addEventListener('scroll', this.handleScroll)
}
destroyed() {
window.removeEventListener('scroll', this.handleScroll)
}
handleScroll() {
const scrollTop = window.pageYOffset || document.documentElement.scrollTop || document.body.scrollTop
// 处理业务逻辑
}
}
</script>
```
vue3+ts 监听怎么写
### 回答1:
在 Vue 3 中使用 TypeScript 监听事件的方法与 Vue 2 类似。首先,在组件中声明事件名称和类型:
```
<template>
<div @eventName="eventHandler"></div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
methods: {
eventHandler(event: Event) {
// event handling logic
},
},
});
</script>
```
在上面代码中,@eventName 是事件名称,"eventHandler" 是事件处理函数。在 eventHandler 函数中,我们可以使用 event 参数来访问事件对象。
注意:如果你使用了 TypeScript,一定要确保你的 event 是正确的类型,如上面代码中的 Event。
### 回答2:
在Vue 3中,使用TypeScript编写监听相对于Vue 2有一些改变。在Vue 3中,我们需要使用`watch`函数来定义监听器。
首先,在Vue组件的`setup`方法中,我们需要使用`watch`函数来定义一个监听器。可以在组件的`setup`方法中使用`watch`来监听特定的数据,一旦数据发生变化,监听器就会自动执行对应的回调函数。
下面是一个使用Vue 3和TypeScript编写的监听器的示例:
```typescript
import { defineComponent, ref, watch } from 'vue';
export default defineComponent({
setup() {
// 定义一个响应式数据
const count = ref(0);
// 监听count的变化
watch(count, (newValue, oldValue) => {
console.log('count发生变化: ' + newValue);
});
// 在组件模板中渲染count
return {
count
};
}
});
```
在上面的示例中,我们使用`ref`函数来创建一个响应式数据`count`,然后使用`watch`函数来监听`count`的变化。当`count`发生变化时,回调函数将被执行,并打印出变化后的新值。最后,在组件模板中渲染`count`。
需要注意的是,`watch`函数的第一个参数可以是一个表达式,也可以是一个返回值为响应式数据的函数。这使得我们可以对多个数据进行监听。
除了基本的监听器,Vue 3还提供了更高级的监听器选项,比如`immediate`、`deep`等。这些选项可以在需要时用来精确地调整监听器的行为。
总之,在Vue 3中,我们可以使用`watch`函数来创建监听器,以便于在响应式数据发生变化时执行相应的逻辑。
### 回答3:
在Vue 3中,我们可以使用TypeScript来编写监听方法。
首先,在Vue组件中,我们可以使用`@Watch`装饰器来声明一个监听方法。在监听方法中,我们可以使用特定的语法来指定要监听的属性和要执行的逻辑。
下面是一个使用Vue 3和TypeScript编写的监听方法的示例:
```typescript
import { Options, Vue } from 'vue-class-component';
@Options({
name: 'my-component',
})
export default class MyComponent extends Vue {
private message: string = 'Hello, World!';
@Watch('message')
onMessageChange(newValue: string, oldValue: string): void {
console.log(`message changed from ${oldValue} to ${newValue}`);
}
}
```
在上面的示例中,我们使用`@Watch`装饰器来定义一个监听方法`onMessageChange`,它监听`message`属性的变化。当`message`属性发生变化时,`onMessageChange`方法会被触发,并将新值和旧值作为参数传递给方法。
通过以上方法,我们可以在Vue 3中使用TypeScript编写监听方法,方便地监听组件中的属性变化,并执行相应的逻辑。
相关推荐
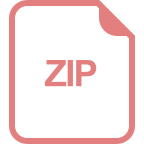
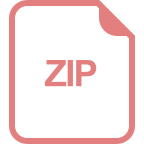
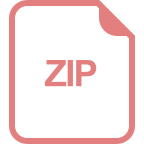
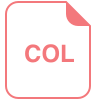











