使用vue写一个购物车代码完整
时间: 2024-02-08 13:21:58 浏览: 140
这里给出一个简单的购物车代码示例,使用Vue 3和Composition API编写。
首先,我们需要创建一个新的Vue实例,并在其中定义一个名为“App”的组件:
```html
<!DOCTYPE html>
<html>
<head>
<title>Vue Shopping Cart</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div id="app">
<app></app>
</div>
<script>
const app = Vue.createApp({
components: {
App: {
template: `
<div>
<h1>Vue Shopping Cart</h1>
<ul>
<li v-for="(product, index) in products" :key="index">
{{ product.name }} - {{ product.price }}
<button @click="addToCart(product)">Add to Cart</button>
</li>
</ul>
<h2>Cart</h2>
<ul>
<li v-for="(item, index) in cart" :key="index">
{{ item.name }} - {{ item.price }} x {{ item.quantity }}
<button @click="removeFromCart(item)">Remove from Cart</button>
</li>
</ul>
</div>
`,
setup() {
const products = Vue.ref([
{ name: 'Product A', price: 10 },
{ name: 'Product B', price: 20 },
{ name: 'Product C', price: 30 },
])
const cart = Vue.reactive([])
const addToCart = (product) => {
const existingItem = cart.find((item) => item.name === product.name)
if (existingItem) {
existingItem.quantity++
} else {
cart.push({ ...product, quantity: 1 })
}
}
const removeFromCart = (item) => {
const index = cart.indexOf(item)
if (index > -1) {
cart.splice(index, 1)
}
}
return { products, cart, addToCart, removeFromCart }
},
},
},
})
app.mount('#app')
</script>
</body>
</html>
```
在上面的代码中,我们使用Vue的Composition API来定义了一些响应式的数据和方法:
- `products`:一个包含所有商品的数组
- `cart`:一个包含所有购物车商品的数组
- `addToCart`:将商品添加到购物车中的方法
- `removeFromCart`:从购物车中删除商品的方法
我们在组件的模板中使用了Vue的指令,例如`v-for`和`@click`,来展示商品列表和购物车列表,并调用相应的方法。
当我们点击“Add to Cart”按钮时,`addToCart`方法会检查购物车中是否已经存在该商品,如果是,则增加该商品的数量,否则将该商品添加到购物车中。当我们点击“Remove from Cart”按钮时,`removeFromCart`方法会从购物车中删除该商品。
这是一个简单的购物车示例,您可以根据您的需求进行更改和扩展。
阅读全文
相关推荐
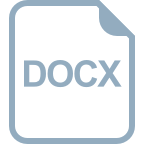
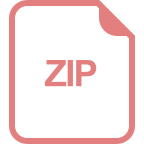
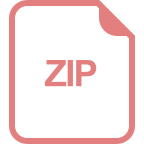
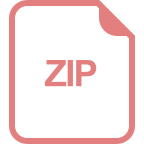
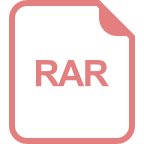
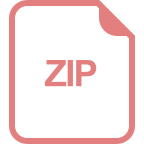
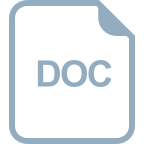
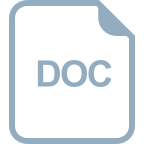
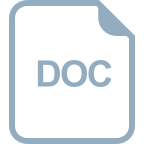
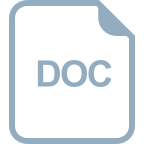


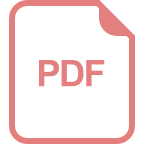
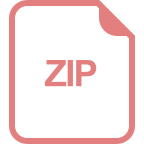