写入的数据使用std::cin实现
时间: 2024-05-03 21:17:22 浏览: 8
好的,那么您可以使用以下代码从标准输入读取数据并将其存储到变量中:
```c++
#include <iostream>
int main() {
int num;
std::cout << "请输入一个整数:";
std::cin >> num;
std::cout << "您输入的整数是:" << num << std::endl;
return 0;
}
```
在上面的代码中,我们使用了 `std::cin` 来读取用户输入的整数,并将其存储到 `num` 变量中。然后,我们使用 `std::cout` 来输出该整数。您可以根据需要修改代码以读取不同类型的数据。
相关问题
std::cin和std::cout和std::endl
std::cin和std::cout都是C++标准库中的流对象,分别用于从标准输入流中读取数据和向标准输出流中写入数据。两者都需要包含头文件iostream来使用。
std::endl是C++标准库中的一个操作符,用于向输出流中插入一个换行符,并刷新输出缓冲区。通常情况下,我们可以使用'\n'来表示换行符,但使用std::endl可以保证在输出换行符后立即刷新缓冲区,确保输出的数据可以及时显示在屏幕上。例如,可以使用std::cout << "Hello world!" << std::endl;输出"Hello world!"并换行。
需要注意的是,std::endl是一个操作符,而不是一个普通的函数或变量,因此不需要使用括号来调用。
QT中使用什么来代替std::cin
在QT中,可以使用QTextStream来代替std::cin。QTextStream是一个用于读取和写入文本的类,可以从标准输入、文件、字符串等多种来源读取数据。使用QTextStream可以方便地进行输入输出操作,而且可以避免一些与std::cin相关的问题。
相关推荐
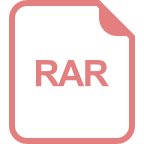
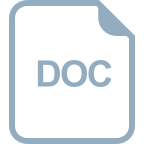
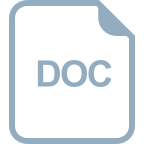












