用异或加密的算法C语言
时间: 2024-05-02 15:16:11 浏览: 104
以下是一个简单的异或加密算法C语言实现:
```c
#include <stdio.h>
#include <string.h>
void xor_encrypt(char *input, char *key, char *output) {
int len = strlen(input);
int keyLen = strlen(key);
for (int i = 0; i < len; i++) {
output[i] = input[i] ^ key[i % keyLen];
}
}
void xor_decrypt(char *input, char *key, char *output) {
int len = strlen(input);
int keyLen = strlen(key);
for (int i = 0; i < len; i++) {
output[i] = input[i] ^ key[i % keyLen];
}
}
int main() {
char input[] = "Hello, world!";
char key[] = "secret";
char encrypted[strlen(input)];
char decrypted[strlen(input)];
xor_encrypt(input, key, encrypted);
printf("Encrypted: %s\n", encrypted);
xor_decrypt(encrypted, key, decrypted);
printf("Decrypted: %s\n", decrypted);
return 0;
}
```
在上面的代码中,`xor_encrypt()` 函数将输入字符串 `input` 和密钥 `key` 异或加密并将结果存储在 `output` 中。`xor_decrypt()` 函数将加密后的字符串 `input` 和密钥 `key` 进行异或解密并将结果存储在 `output` 中。在主函数中,我们使用这两个函数来加密和解密字符串。
阅读全文
相关推荐
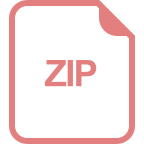
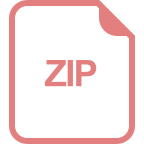
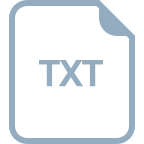















