python连接数据库
时间: 2023-06-30 22:04:02 浏览: 120
Python 可以通过多种方式连接数据库,以下是其中两种常见的方式:
1. 使用 Python 官方提供的 DB-API
Python 标准库中提供了 DB-API 规范,可以使用该规范连接主流的数据库,如 MySQL、Oracle、PostgreSQL、SQLite 等。下面以 MySQL 为例,介绍如何使用 DB-API 连接 MySQL 数据库:
```python
import mysql.connector
# 创建数据库连接
cnx = mysql.connector.connect(user='root', password='password', host='localhost', database='test')
# 创建游标对象
cursor = cnx.cursor()
# 执行 SQL 查询
query = ("SELECT id, name FROM users")
cursor.execute(query)
# 处理查询结果
for (id, name) in cursor:
print("User id={}, name={}".format(id, name))
# 关闭游标和数据库连接
cursor.close()
cnx.close()
```
2. 使用 ORM 框架
ORM(Object-Relational Mapping)是一种将关系型数据库映射到对象的编程技术,可以方便地操作数据库。Python 中有多种 ORM 框架可供选择,如 Django ORM、SQLAlchemy、Peewee 等。下面以 SQLAlchemy 为例,介绍如何使用 SQLAlchemy 连接 MySQL 数据库:
```python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
# 创建数据库连接
engine = create_engine('mysql+mysqlconnector://root:password@localhost:3306/test')
# 创建会话工厂类
Session = sessionmaker(bind=engine)
# 创建基类
Base = declarative_base()
# 定义数据表模型
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String(50))
# 创建会话对象
session = Session()
# 查询数据
users = session.query(User).all()
for user in users:
print("User id={}, name={}".format(user.id, user.name))
# 关闭会话对象
session.close()
```
以上是两种常见的连接数据库的方式,具体使用哪种方式取决于项目需求和个人习惯。
阅读全文
相关推荐
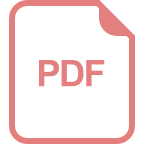
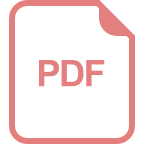
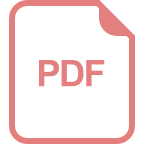
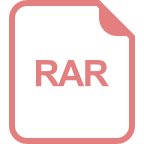
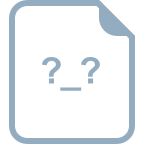
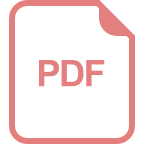
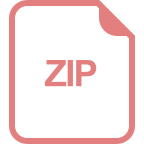
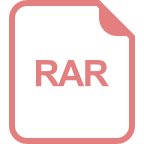
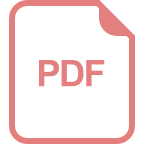
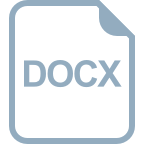
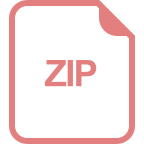
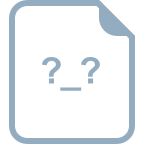
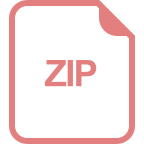