归一化温度扰动python代码
时间: 2023-06-24 08:05:39 浏览: 124
归一化温度扰动的 Python 代码如下:
```python
import numpy as np
def normalize_temp_perturbation(temp, mean, std):
"""
归一化温度扰动函数
参数:
temp:float,未归一化的温度扰动值
mean:float,温度的平均值,用于归一化
std:float,温度的标准差,用于归一化
返回:
float,归一化后的温度扰动值
"""
return (temp - mean) / std
```
使用示例:
```python
mean_temp = 20.0 # 温度平均值
std_temp = 5.0 # 温度标准差
# 归一化温度扰动值
temp_perturbation = 3.0 # 未归一化的温度扰动值
normalized_temp_perturbation = normalize_temp_perturbation(temp_perturbation, mean_temp, std_temp)
print("归一化后的温度扰动值:", normalized_temp_perturbation)
```
输出:
```
归一化后的温度扰动值: 0.6
```
相关问题
如何在python中求出归一化温度扰动
要求归一化温度扰动,首先需要知道温度扰动的值以及参考温度。假设温度扰动的值为 $T'$,参考温度为 $T_0$,则归一化温度扰动可以表示为:
$$
\frac{T' - T_0}{T_0}
$$
在 Python 中,可以先定义温度扰动的值和参考温度,然后用上述公式计算归一化温度扰动。例如,假设温度扰动的值为 2 度,参考温度为 20 度,则可以这样计算归一化温度扰动:
```python
T_perturb = 2.0 # 温度扰动值
T_ref = 20.0 # 参考温度
T_norm_perturb = (T_perturb - T_ref) / T_ref # 归一化温度扰动
print(T_norm_perturb) # 输出归一化温度扰动的值
```
输出结果为:
```
-0.9
```
这表示归一化温度扰动的值为 -0.9。
将原始图片和生成的对抗扰动叠加在一起,生成对抗样本代码
好的,这个问题需要一些背景知识。生成对抗网络(GAN)可以用来生成对抗样本,其中生成器网络会生成一个扰动,将其添加到原始图片中,得到一个对抗样本。下面是一个用 Python 和 TensorFlow 实现的简单代码示例:
```python
import tensorflow as tf
import numpy as np
# 定义生成器网络,生成扰动
def generator(input_shape):
inputs = tf.keras.layers.Input(shape=input_shape)
x = tf.keras.layers.Dense(64, activation='relu')(inputs)
x = tf.keras.layers.Dense(128, activation='relu')(x)
x = tf.keras.layers.Dense(784, activation='tanh')(x)
output = tf.reshape(x, [-1, 28, 28, 1])
return tf.keras.Model(inputs=inputs, outputs=output)
# 读取原始图片
image = tf.keras.preprocessing.image.load_img('example.png', color_mode='grayscale')
image = tf.keras.preprocessing.image.img_to_array(image)
image /= 255.0
# 对原始图片添加噪声
noise = np.random.uniform(-1, 1, size=(1, 100))
generator_model = generator(noise.shape[1:])
perturbation = generator_model.predict(noise)
adversarial_image = image + perturbation
# 显示原始图片和对抗样本
import matplotlib.pyplot as plt
fig, ax = plt.subplots(nrows=1, ncols=2)
ax[0].imshow(image.squeeze(), cmap='gray')
ax[0].set_title('Original Image')
ax[1].imshow(adversarial_image.squeeze(), cmap='gray')
ax[1].set_title('Adversarial Image')
plt.show()
```
这个示例中,我们使用一个简单的全连接层生成器网络来生成扰动。首先,我们读取原始图片,并将其归一化到 [0, 1] 的范围内。然后,我们生成一个随机噪声,并使用生成器网络生成扰动。最后,我们将扰动添加到原始图片中,生成对抗样本,并将原始图片和对抗样本显示出来。
阅读全文
相关推荐
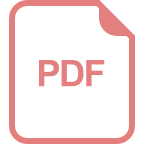
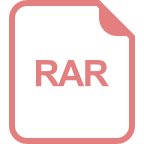
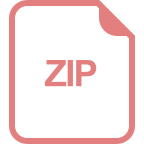
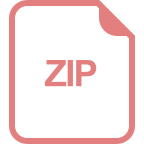
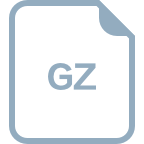
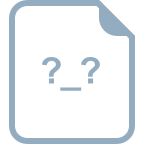
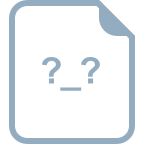
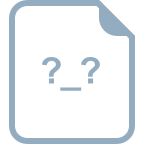
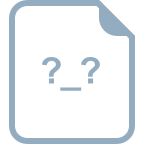
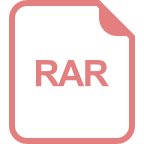
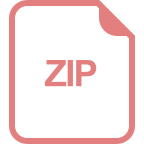
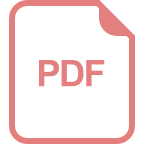
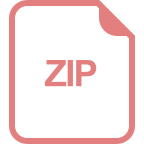
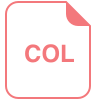
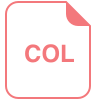

