1、 完成学生信息管理系统中的注册和验证页面。 要求: a)注册页面中需要输入用户名、密码、确认密码以及身份的选择和验证码的输入。 b) 注册页面中的每个输入均需判断是否为空,为空进行提示。 C) 注册的用户名必须是从未有人注册的,如已有,请提示:“用户名已存在!” d)用户名必须满足当身份为教师时长度为5位,当身份为学生时长度为9位,当身份为管理员时长度为13位。 e)密码和确认密码必须保持一致,否则,请提示:“两次输入的密码不一致!”。 f) 验证码为4位随机数字,当输入不正确时,请提示:“验证码输入错误!” g) 以上条件均满足,请跳转至登录页面。 h) 数据库请自行设计。的代码
时间: 2024-03-08 07:46:43 浏览: 121
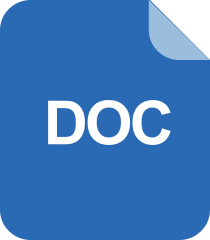
学生信息管理学生信息包括:学号,姓名,年龄,性别,出生年月,地址,电话,E-mail等。设计“学生信息管理系统”

好的,下面是一个简单的实现示例,仅供参考:
HTML代码:
```
<form action="register.php" method="post">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<label for="confirm_password">确认密码:</label>
<input type="password" id="confirm_password" name="confirm_password"><br>
<label for="identity">身份:</label>
<select id="identity" name="identity">
<option value="student">学生</option>
<option value="teacher">教师</option>
<option value="admin">管理员</option>
</select><br>
<label for="captcha">验证码:</label>
<input type="text" id="captcha" name="captcha"><br>
<img src="captcha.php" alt="验证码"><br>
<input type="submit" value="注册">
</form>
```
PHP代码(register.php):
```
<?php
// 连接数据库
$dbc = mysqli_connect('localhost', 'username', 'password', 'database_name')
or die('错误:无法连接到数据库');
// 获取表单数据
$username = mysqli_real_escape_string($dbc, trim($_POST['username']));
$password = mysqli_real_escape_string($dbc, trim($_POST['password']));
$confirm_password = mysqli_real_escape_string($dbc, trim($_POST['confirm_password']));
$identity = mysqli_real_escape_string($dbc, trim($_POST['identity']));
$captcha = mysqli_real_escape_string($dbc, trim($_POST['captcha']));
// 检查输入是否为空
if (empty($username) || empty($password) || empty($confirm_password) || empty($identity) || empty($captcha)) {
echo '错误:请填写所有字段!';
exit();
}
// 检查用户名长度
if (($identity == 'student' && strlen($username) != 9) ||
($identity == 'teacher' && strlen($username) != 5) ||
($identity == 'admin' && strlen($username) != 13)) {
echo '错误:用户名长度不正确!';
exit();
}
// 检查密码是否一致
if ($password != $confirm_password) {
echo '错误:两次输入的密码不一致!';
exit();
}
// 检查验证码是否正确
session_start();
if ($captcha != $_SESSION['captcha']) {
echo '错误:验证码输入错误!';
exit();
}
// 检查用户名是否已存在
$query = "SELECT * FROM users WHERE username = '$username'";
$result = mysqli_query($dbc, $query);
if (mysqli_num_rows($result) != 0) {
echo '错误:用户名已存在!';
exit();
}
// 将用户信息插入数据库
$query = "INSERT INTO users (username, password, identity) VALUES ('$username', SHA('$password'), '$identity')";
mysqli_query($dbc, $query)
or die('错误:无法插入数据!');
// 跳转至登录页面
header('Location: login.php');
mysqli_close($dbc);
?>
```
PHP代码(captcha.php):
```
<?php
session_start();
$rand_num = rand(1000, 9999);
$_SESSION['captcha'] = $rand_num;
// 生成验证码图片
$captcha_image = imagecreate(60, 30);
$bg_color = imagecolorallocate($captcha_image, 255, 255, 255);
$text_color = imagecolorallocate($captcha_image, 0, 0, 0);
imagestring($captcha_image, 5, 10, 5, $rand_num, $text_color);
header('Content-Type: image/png');
imagepng($captcha_image);
imagedestroy($captcha_image);
?>
```
这是一个基础的实现,实际上还有很多细节需要考虑,比如密码加密、验证码的安全性等等。此外,数据库的设计也需要根据实际需求进行,这里只是提供一个简单的示例。
阅读全文
相关推荐
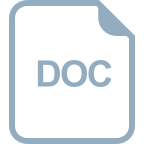
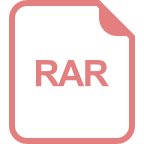
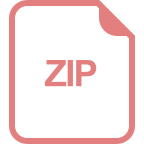
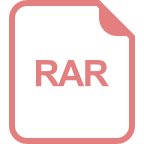
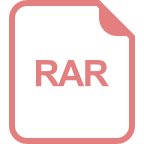
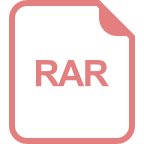
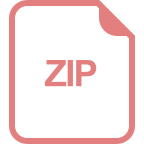
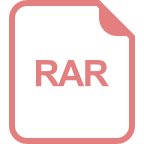
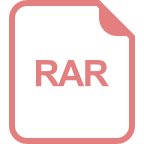
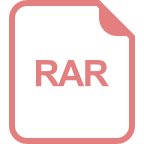
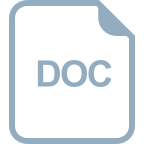
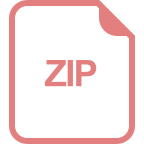
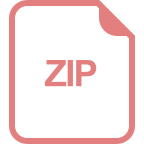
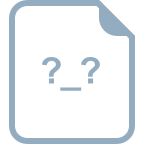
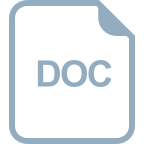
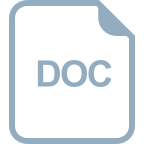